jigdo API
mkimage.cc File Reference
#include <config.h>
#include <errno.h>
#include <stdio.h>
#include <string.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd-jigdo.h>
#include <iomanip>
#include <iostream>
#include <fstream>
#include <memory>
#include <compat.hh>
#include <log.hh>
#include <mkimage.hh>
#include <scan.hh>
#include <serialize.hh>
#include <string.hh>
#include <zstream-gz.hh>
Typedefs | |
typedef JigdoDesc::ProgressReporter | ProgressReporter |
Enumerations | |
enum | Task { CREATE_TMP, MERGE_TMP, SINGLE_PASS } |
Type of operation when recreating image data. More... | |
Functions | |
void | memClear (byte *buf, size_t size) |
void | reportBytesWritten (const uint64 n, uint64 &off, uint64 &nextReport, const uint64 totalBytes, ProgressReporter &reporter) |
int | fileToImage (bostream *img, FilePart &file, const JigdoDesc::MatchedFile &matched, bool checkMD5, size_t rsyncLen, ProgressReporter &reporter, byte *buf, size_t readAmount, uint64 &off, uint64 &nextReport, const uint64 totalBytes) |
int | writeAll (const Task &task, JigdoDescVec &files, queue< FilePart * > &toCopy, bistream *templ, const size_t readAmount, bostream *img, const char *name, bool checkMD5, ProgressReporter &reporter, JigdoCache *cache, const uint64 totalBytes) |
int | writeMerge (JigdoDescVec &files, queue< FilePart * > &toCopy, const int missing, const size_t readAmount, bfstream *img, const string &imageTmpFile, bool checkMD5, ProgressReporter &reporter, JigdoCache *cache, const uint64 totalBytes) |
int | info_NeedMoreFiles (ProgressReporter &reporter, const string &tmpName) |
int | error_CouldntRename (ProgressReporter &reporter, const char *name, const char *finalName) |
void | readTemplate (JigdoDescVec &files, const string &templFile, bistream *templ) |
Read template data from templ (name in templFile) into files. | |
const char * | readTmpFile (bistream &imageTmp, JigdoDescVec &filesTmp, const JigdoDescVec &files) |
Read data from end of temporary file imageTmp, output it to filesTmp. | |
Variables | |
const int | SIZE_WIDTH = 12 |
Typedef Documentation
typedef JigdoDesc::ProgressReporter ProgressReporter [static] |
Enumeration Type Documentation
enum Task |
Function Documentation
int @53::error_CouldntRename | ( | ProgressReporter & | reporter, | |
const char * | name, | |||
const char * | finalName | |||
) | [static] |
References _, JigdoDesc::ProgressReporter::error(), and subst.
Referenced by JigdoDesc::makeImage().
int @53::fileToImage | ( | bostream * | img, | |
FilePart & | file, | |||
const JigdoDesc::MatchedFile & | matched, | |||
bool | checkMD5, | |||
size_t | rsyncLen, | |||
ProgressReporter & | reporter, | |||
byte * | buf, | |||
size_t | readAmount, | |||
uint64 & | off, | |||
uint64 & | nextReport, | |||
const uint64 | totalBytes | |||
) | [static] |
References _, RsyncSum64::addBack(), JigdoDesc::ProgressReporter::error(), MD5Sum::finish(), FilePart::getPath(), FilePart::leafName(), md, JigdoDesc::MatchedFile::md5(), memClear(), Paranoid, readBytes(), reportBytesWritten(), JigdoDesc::MatchedFile::rsync(), FilePart::size(), subst, MD5Sum::update(), and writeBytes().
Referenced by writeAll(), and writeMerge().
int @53::info_NeedMoreFiles | ( | ProgressReporter & | reporter, | |
const string & | tmpName | |||
) | [static] |
References _, JigdoDesc::ProgressReporter::info(), info, and subst.
Referenced by JigdoDesc::makeImage().
void @53::memClear | ( | byte * | buf, | |
size_t | size | |||
) | [static] |
Referenced by fileToImage(), and writeAll().
void @53::readTemplate | ( | JigdoDescVec & | files, | |
const string & | templFile, | |||
bistream * | templ | |||
) | [static] |
Read template data from templ (name in templFile) into files.
References _, JigdoDesc::isTemplate(), JigdoDesc::seekFromEnd(), and subst.
Referenced by JigdoDesc::listMissing(), and JigdoDesc::makeImage().
const char* @53::readTmpFile | ( | bistream & | imageTmp, | |
JigdoDescVec & | filesTmp, | |||
const JigdoDescVec & | files | |||
) | [static] |
Read data from end of temporary file imageTmp, output it to filesTmp.
Next, compare it to template data in "files". If tmp file is OK for re-using return NULL - this means that the DESC entries match *exactly* - the only difference allowed is MatchedFile turning into WrittenFile. Otherwise, return a pointer to an error message describing the reason why the tmpfile data does not match the template data.
References _, and JigdoDesc::seekFromEnd().
Referenced by JigdoDesc::listMissing(), and JigdoDesc::makeImage().
void @53::reportBytesWritten | ( | const uint64 | n, | |
uint64 & | off, | |||
uint64 & | nextReport, | |||
const uint64 | totalBytes, | |||
ProgressReporter & | reporter | |||
) | [inline, static] |
References JigdoDesc::ProgressReporter::writingImage().
Referenced by fileToImage(), and writeAll().
int @53::writeAll | ( | const Task & | task, | |
JigdoDescVec & | files, | |||
queue< FilePart * > & | toCopy, | |||
bistream * | templ, | |||
const size_t | readAmount, | |||
bostream * | img, | |||
const char * | name, | |||
bool | checkMD5, | |||
ProgressReporter & | reporter, | |||
JigdoCache * | cache, | |||
const uint64 | totalBytes | |||
) | [inline, static] |
References _, Assert, JigdoDesc::ImageInfo::blockLength(), buf, CREATE_TMP, data, debug, JigdoDesc::ProgressReporter::error(), fileToImage(), FilePart::getMD5Sum(), JigdoDesc::IMAGE_INFO, JigdoDesc::isTemplate(), FilePart::leafName(), JigdoDesc::MATCHED_FILE, memClear(), Error::message, JigdoDesc::OBSOLETE_IMAGE_INFO, JigdoDesc::OBSOLETE_MATCHED_FILE, JigdoDesc::OBSOLETE_WRITTEN_FILE, off, reportBytesWritten(), SINGLE_PASS, FilePart::size(), subst, JigdoDesc::UNMATCHED_DATA, writeBytes(), and JigdoDesc::WRITTEN_FILE.
Referenced by JigdoDesc::makeImage().
int @53::writeMerge | ( | JigdoDescVec & | files, | |
queue< FilePart * > & | toCopy, | |||
const int | missing, | |||
const size_t | readAmount, | |||
bfstream * | img, | |||
const string & | imageTmpFile, | |||
bool | checkMD5, | |||
ProgressReporter & | reporter, | |||
JigdoCache * | cache, | |||
const uint64 | totalBytes | |||
) | [inline, static] |
Variable Documentation
const int SIZE_WIDTH = 12 [static] |
Generated on Tue Sep 23 14:27:42 2008 for jigdo by
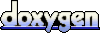