jigdo API
Last update by Admin on 2010-05-23
mkimage.hh
Go to the documentation of this file.00001 /* $Id: mkimage.hh,v 1.26 2003/03/03 20:47:17 richard Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2001-2002 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00016 #ifndef MKIMAGE_HH 00017 #define MKIMAGE_HH 00018 00019 #include <config.h> 00020 00021 #include <iosfwd> 00022 #include <queue> 00023 #include <vector> 00024 #include <typeinfo> 00025 00026 #include <bstream.hh> 00027 #include <debug.hh> 00028 #include <md5sum.hh> 00029 #include <scan.hh> 00030 #include <serialize.hh> 00031 //______________________________________________________________________ 00032 00034 struct JigdoDescError : Error { 00035 explicit JigdoDescError(const string& m) : Error(m) { } 00036 explicit JigdoDescError(const char* m) : Error(m) { } 00037 }; 00038 00042 class JigdoDesc { 00043 public: 00045 enum Type { 00046 IMAGE_INFO = 5, UNMATCHED_DATA = 2, MATCHED_FILE = 6, WRITTEN_FILE = 7, 00047 OBSOLETE_IMAGE_INFO = 1, OBSOLETE_MATCHED_FILE = 3, 00048 OBSOLETE_WRITTEN_FILE = 4 00049 }; 00050 class ProgressReporter; 00051 //____________________ 00052 00053 virtual bool operator==(const JigdoDesc& x) const = 0; 00054 inline bool operator!=(const JigdoDesc& x) const { return !(*this == x); } 00055 virtual ~JigdoDesc() = 0; 00056 00058 virtual Type type() const = 0; 00060 virtual ostream& put(ostream& s) const = 0; 00062 virtual uint64 size() const = 0; 00063 00067 virtual size_t serialSizeOf() const = 0; 00068 00071 static bool isTemplate(bistream& file); 00075 static void seekFromEnd(bistream& file) throw(JigdoDescError); 00077 static int makeImage(JigdoCache* cache, const string& imageFile, 00078 const string& imageTmpFile, const string& templFile, 00079 bistream* templ, const bool optForce, 00080 ProgressReporter& pr = noReport, size_t readAmnt = 128U*1024, 00081 const bool optMkImageCheck = true); 00086 static int listMissing(set<MD5>& result, const string& imageTmpFile, 00087 const string& templFile, bistream* templ, ProgressReporter& reporter); 00088 00089 class ImageInfo; 00090 class UnmatchedData; 00091 class MatchedFile; 00092 class WrittenFile; 00093 00094 private: 00095 static ProgressReporter noReport; 00096 }; 00097 00098 inline ostream& operator<<(ostream& s, JigdoDesc& jd) { return jd.put(s); } 00099 //______________________________________________________________________ 00100 00102 class JigdoDesc::ImageInfo : public JigdoDesc { 00103 public: 00104 inline ImageInfo(uint64 s, const MD5& m, size_t b); 00105 inline ImageInfo(uint64 s, const MD5Sum& m, size_t b); 00106 inline bool operator==(const JigdoDesc& x) const; 00107 Type type() const { return IMAGE_INFO; } 00108 uint64 size() const { return sizeVal; } 00109 const MD5& md5() const { return md5Val; } 00110 size_t blockLength() const { return blockLengthVal; } 00111 // Default dtor, operator== 00112 virtual ostream& put(ostream& s) const; 00113 00114 template<class Iterator> 00115 inline Iterator serialize(Iterator i) const; 00116 inline size_t serialSizeOf() const; 00117 00118 private: 00119 uint64 sizeVal; 00120 MD5 md5Val; 00121 size_t blockLengthVal; 00122 }; 00123 //________________________________________ 00124 00127 class JigdoDesc::UnmatchedData : public JigdoDesc { 00128 public: 00129 UnmatchedData(uint64 o, uint64 s) : offsetVal(o), sizeVal(s) { } 00130 inline bool operator==(const JigdoDesc& x) const; 00131 Type type() const { return UNMATCHED_DATA; } 00132 uint64 offset() const { return offsetVal; } 00133 uint64 size() const { return sizeVal; } 00134 void resize(uint64 s) { sizeVal = s; } 00135 // Default dtor, operator== 00136 virtual ostream& put(ostream& s) const; 00137 00138 template<class Iterator> 00139 inline Iterator serialize(Iterator i) const; 00140 inline size_t serialSizeOf() const; 00141 00142 private: 00143 uint64 offsetVal; // Offset in image 00144 uint64 sizeVal; 00145 }; 00146 //________________________________________ 00147 00149 class JigdoDesc::MatchedFile : public JigdoDesc { 00150 public: 00151 inline MatchedFile(uint64 o, uint64 s, const RsyncSum64& r, const MD5& m); 00152 inline MatchedFile(uint64 o, uint64 s, const RsyncSum64& r, 00153 const MD5Sum& m); 00154 inline bool operator==(const JigdoDesc& x) const; 00155 Type type() const { return MATCHED_FILE; } 00156 uint64 offset() const { return offsetVal; } 00157 uint64 size() const { return sizeVal; } 00158 const MD5& md5() const { return md5Val; } 00159 const RsyncSum64& rsync() const { return rsyncVal; } 00160 // Default dtor, operator== 00161 virtual ostream& put(ostream& s) const; 00162 00163 template<class Iterator> 00164 inline Iterator serialize(Iterator i) const; 00165 inline size_t serialSizeOf() const; 00166 00167 private: 00168 uint64 offsetVal; // Offset in image 00169 uint64 sizeVal; 00170 RsyncSum64 rsyncVal; 00171 MD5 md5Val; 00172 }; 00173 //________________________________________ 00174 00180 class JigdoDesc::WrittenFile : public MatchedFile { 00181 public: 00182 WrittenFile(uint64 o, uint64 s, const RsyncSum64& r, const MD5& m) 00183 : MatchedFile(o, s, r, m) { } 00184 // Implicit cast to allow MatchedFile and WrittenFile to compare equal 00185 inline bool operator==(const JigdoDesc& x) const; 00186 Type type() const { return WRITTEN_FILE; } 00187 virtual ostream& put(ostream& s) const; 00188 00189 template<class Iterator> 00190 inline Iterator serialize(Iterator i) const; 00191 inline size_t serialSizeOf() const; 00192 }; 00193 //______________________________________________________________________ 00194 00199 class JigdoDesc::ProgressReporter { 00200 public: 00201 virtual ~ProgressReporter() { } 00203 virtual void error(const string& message); 00205 virtual void info(const string& message); 00214 virtual void writingImage(uint64 written, uint64 totalToWrite, 00215 uint64 imgOff, uint64 imgSize); 00216 }; 00217 //______________________________________________________________________ 00218 00223 class JigdoDescVec : public vector<JigdoDesc*> { 00224 public: 00225 JigdoDescVec() : vector<JigdoDesc*>() { } 00226 inline ~JigdoDescVec(); 00227 00233 bistream& get(bistream& file) throw(JigdoDescError, bad_alloc); 00234 00239 bostream& put(bostream& file, MD5Sum* md = 0) const; 00240 00242 void list(ostream& s) throw(); 00243 private: 00244 // Disallow copying (too inefficient). Use swap() instead. 00245 inline JigdoDescVec& operator=(const JigdoDescVec&); 00246 }; 00247 00248 inline void swap(JigdoDescVec& x, JigdoDescVec& y) { x.swap(y); } 00249 00250 //====================================================================== 00251 00252 JigdoDesc::ImageInfo::ImageInfo(uint64 s, const MD5& m, size_t b) 00253 : sizeVal(s), md5Val(m), blockLengthVal(b) { } 00254 JigdoDesc::ImageInfo::ImageInfo(uint64 s, const MD5Sum& m, size_t b) 00255 : sizeVal(s), md5Val(m), blockLengthVal(b) { } 00256 00257 JigdoDesc::MatchedFile::MatchedFile(uint64 o, uint64 s, const RsyncSum64& r, 00258 const MD5& m) 00259 : offsetVal(o), sizeVal(s), rsyncVal(r), md5Val(m) { } 00260 JigdoDesc::MatchedFile::MatchedFile(uint64 o, uint64 s, const RsyncSum64& r, 00261 const MD5Sum& m) 00262 : offsetVal(o), sizeVal(s), rsyncVal(r), md5Val(m) { } 00263 00264 //________________________________________ 00265 00266 bool JigdoDesc::ImageInfo::operator==(const JigdoDesc& x) const { 00267 const ImageInfo* i = dynamic_cast<const ImageInfo*>(&x); 00268 if (i == 0) return false; 00269 else return size() == i->size() && md5() == i->md5(); 00270 } 00271 00272 bool JigdoDesc::UnmatchedData::operator==(const JigdoDesc& x) const { 00273 const UnmatchedData* u = dynamic_cast<const UnmatchedData*>(&x); 00274 if (u == 0) return false; 00275 else return size() == u->size(); 00276 } 00277 00278 bool JigdoDesc::MatchedFile::operator==(const JigdoDesc& x) const { 00279 const MatchedFile* m = dynamic_cast<const MatchedFile*>(&x); 00280 if (m == 0) return false; 00281 else return offset() == m->offset() && size() == m->size() 00282 && md5() == m->md5(); 00283 } 00284 00285 bool JigdoDesc::WrittenFile::operator==(const JigdoDesc& x) const { 00286 // NB MatchedFile and WrittenFile considered equal! 00287 const MatchedFile* m = dynamic_cast<const MatchedFile*>(&x); 00288 if (m == 0) return false; 00289 else return offset() == m->offset() && size() == m->size() 00290 && md5() == m->md5(); 00291 } 00292 //________________________________________ 00293 00294 inline bistream& operator>>(bistream& s, JigdoDescVec& v) 00295 throw(JigdoDescError, bad_alloc) { 00296 return v.get(s); 00297 } 00298 00299 inline bostream& operator<<(bostream& s, JigdoDescVec& v) { 00300 return v.put(s); 00301 } 00302 00303 JigdoDescVec::~JigdoDescVec() { 00304 for (iterator i = begin(), e = end(); i != e; ++i) delete *i; 00305 } 00306 //________________________________________ 00307 00308 template<class Iterator> 00309 Iterator JigdoDesc::ImageInfo::serialize(Iterator i) const { 00310 i = serialize1(IMAGE_INFO, i); 00311 i = serialize6(size(), i); 00312 i = ::serialize(md5(), i); 00313 i = serialize4(blockLength(), i); 00314 return i; 00315 } 00316 size_t JigdoDesc::ImageInfo::serialSizeOf() const { return 1 + 6 + 16 + 4; } 00317 00318 template<class Iterator> 00319 Iterator JigdoDesc::UnmatchedData::serialize(Iterator i) const { 00320 i = serialize1(UNMATCHED_DATA, i); 00321 i = serialize6(size(), i); 00322 return i; 00323 } 00324 size_t JigdoDesc::UnmatchedData::serialSizeOf() const { return 1 + 6; } 00325 00326 template<class Iterator> 00327 Iterator JigdoDesc::MatchedFile::serialize(Iterator i) const { 00328 i = serialize1(MATCHED_FILE, i); 00329 i = serialize6(size(), i); 00330 i = ::serialize(rsync(), i); 00331 i = ::serialize(md5(), i); 00332 return i; 00333 } 00334 size_t JigdoDesc::MatchedFile::serialSizeOf() const { return 1 + 6 + 8 + 16;} 00335 00336 template<class Iterator> 00337 Iterator JigdoDesc::WrittenFile::serialize(Iterator i) const { 00338 i = serialize1(WRITTEN_FILE, i); 00339 i = serialize6(size(), i); 00340 i = ::serialize(rsync(), i); 00341 i = ::serialize(md5(), i); 00342 return i; 00343 } 00344 size_t JigdoDesc::WrittenFile::serialSizeOf() const { return 1 + 6 + 8 + 16;} 00345 00346 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
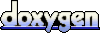