jigdo API
Last update by Admin on 2010-05-23
util/bstream.hh
Go to the documentation of this file.00001 /* $Id: bstream.hh,v 1.1.1.1 2003/07/04 22:30:22 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2001-2004 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00023 #ifndef BSTREAM_HH 00024 #define BSTREAM_HH 00025 00026 #include <config.h> 00027 00028 //#include <iosfwd> /* Needed for fstream prototypes! */ 00029 #include <iostream> 00030 #include <fstream> 00031 00032 #include <stdio.h> 00033 00034 #include <debug.hh> 00035 //____________________ 00036 00037 #if HAVE_WORKING_FSTREAM 00038 00039 typedef istream bistream; 00040 typedef ostream bostream; 00041 typedef iostream biostream; 00042 00043 typedef ifstream bifstream; 00044 typedef ofstream bofstream; 00045 typedef fstream bfstream; 00046 00047 #else 00048 00049 //____________________ 00050 00051 /* With GCC 3.x (x < 4), libstdc++ is broken for files >2GB. We need to 00052 re-implement the basic functionality to work around this. :-/ */ 00053 #if !defined _FILE_OFFSET_BITS || _FILE_OFFSET_BITS != 64 00054 # warning "_FILE_OFFSET_BITS not defined, big file support may not work" 00055 #endif 00056 00057 /* The subtle differences between bad() and fail() are not taken into account 00058 here - beware! */ 00059 class bios { 00060 public: 00061 operator void*() const { return fail() ? (void*)0 : (void*)(-1); } 00062 int operator!() const { return fail(); } 00063 bool fail() const { return f == 0 || feof(f) != 0 || ferror(f) != 0; } 00064 //Incorrect: bool bad() const { return fail(); } 00065 bool eof() const { return f == 0 || feof(f) != 0; } 00066 bool good() const { return !fail(); } // Incorrect? 00067 void close() { if (f != 0) fclose(f); f = 0; } 00068 ~bios() { if (f != 0) fclose(f); } 00069 protected: 00070 bios() : f(0) { } 00071 bios(FILE* stream) : f(stream) { } 00072 FILE* f; 00073 }; 00074 00075 class bostream : virtual public bios { 00076 public: 00077 inline int put(int c) { return putc(c, f); } 00078 bostream& seekp(off_t off, ios::seekdir dir = ios::beg); 00079 inline bostream& write(const char* p, streamsize n); 00080 bostream(FILE* stream) : bios(stream) { } 00081 protected: 00082 bostream() { } 00083 }; 00084 00085 class bistream : virtual public bios { 00086 public: 00087 inline int get(); 00088 inline int peek(); 00089 bistream& seekg(off_t off, ios::seekdir dir = ios::beg); 00090 inline uint64 tellg() const; 00091 void getline(string& l); 00092 inline bistream& read(char* p, streamsize n); 00093 inline uint64 gcount() { uint64 r = gcountVal; gcountVal = 0; return r; } 00094 inline void sync() const { } // NOP 00095 bistream(FILE* stream) : bios(stream) { } 00096 protected: 00097 bistream() : gcountVal(0) { } 00098 private: 00099 uint64 gcountVal; 00100 }; 00101 00102 extern bistream bcin; 00103 extern bostream bcout; 00104 00105 class biostream : virtual public bistream, virtual public bostream { 00106 protected: 00107 biostream() : bistream(), bostream() { } 00108 biostream(FILE* stream) : bistream(stream), bostream(stream) { } 00109 }; 00110 //____________________ 00111 00112 class bifstream : public bistream { 00113 public: 00114 bifstream(FILE* stream) : bistream(stream) { Paranoid(stream == stdin); } 00115 inline bifstream(const char* name, ios::openmode m = ios::in); 00116 inline void open(const char* name, ios::openmode m = ios::in); 00117 }; 00118 00119 class bofstream : public bostream { 00120 public: 00121 bofstream(FILE* stream) : bostream(stream) { Paranoid(stream == stdout); } 00122 inline bofstream(const char* name, ios::openmode m = ios::out); 00123 void open(const char* name, ios::openmode m = ios::out); 00124 }; 00125 00126 class bfstream : public biostream { 00127 public: 00128 bfstream(const char* path, ios::openmode); 00129 }; 00130 //____________________ 00131 00132 int bistream::get() { 00133 int r = getc(f); 00134 gcountVal = (r >= 0 ? 1 : 0); 00135 return r; 00136 } 00137 00138 int bistream::peek() { 00139 int r = getc(f); 00140 gcountVal = (r >= 0 ? 1 : 0); 00141 ungetc(r, f); 00142 return r; 00143 } 00144 00145 uint64 bistream::tellg() const { 00146 return ftello(f); 00147 } 00148 00149 bostream& bostream::write(const char* p, streamsize n) { 00150 Paranoid(f != 0); 00151 fwrite(p, 1, n, f); 00152 return *this; 00153 } 00154 00155 bistream& bistream::read(char* p, streamsize n) { 00156 gcountVal = fread(p, 1, n, f); 00157 return *this; 00158 } 00159 00160 inline void getline(bistream& bi, string& l) { 00161 bi.getline(l); 00162 } 00163 00164 bifstream::bifstream(const char* name, ios::openmode m) : bistream() { 00165 open(name, m); 00166 } 00167 00168 void bifstream::open(const char* name, ios::openmode DEBUG_ONLY_PARAM(m)) { 00169 Paranoid((m & ios::binary) != 0 && f == 0); 00170 f = fopen(name, "rb"); 00171 } 00172 00173 bofstream::bofstream(const char* name, ios::openmode m) : bostream() { 00174 // When opened this way, any existing file is truncated 00175 open(name, m | ios::trunc); 00176 } 00177 00178 #endif /* HAVE_WORKING_FSTREAM */ 00179 //______________________________________________________________________ 00180 00181 // Avoid lots of ugly reinterpret_casts in the code itself 00182 inline bistream& readBytes(bistream& s, byte* buf, streamsize count) { 00183 return s.read(reinterpret_cast<char*>(buf), count); 00184 } 00185 00186 inline biostream& readBytes(biostream& s, byte* buf, streamsize count) { 00187 s.read(reinterpret_cast<char*>(buf), count); 00188 return s; 00189 } 00190 00191 inline bostream& writeBytes(bostream& s, const byte* buf, streamsize count) { 00192 return s.write(reinterpret_cast<const char*>(buf), count); 00193 } 00194 00195 inline biostream& writeBytes(biostream& s, const byte* buf, 00196 streamsize count) { 00197 s.write(reinterpret_cast<const char*>(buf), count); 00198 return s; 00199 } 00200 00201 #endif /* BSTREAM_HH */
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
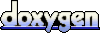