jigdo API
Last update by Admin on 2010-05-23
serialize.hh
Go to the documentation of this file.00001 /* $Id: serialize.hh,v 1.7 2003/03/03 20:47:18 richard Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2001-2002 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00031 #ifndef SERIALIZE_HH 00032 #define SERIALIZE_HH 00033 00034 #include <bstream.hh> 00035 #include <config.h> /* byte, size_t */ 00036 //______________________________________________________________________ 00037 00046 template<class Object, class Iterator> 00047 inline Iterator serialize(const Object& o, Iterator i) { 00048 return o.serialize(i); 00049 } 00050 00053 template<class Object, class ConstIterator> 00054 inline ConstIterator unserialize(Object& o, ConstIterator i) { 00055 return o.unserialize(i); 00056 } 00057 00061 template<class Object> 00062 inline size_t serialSizeOf(const Object& o) { 00063 return o.serialSizeOf(); 00064 } 00065 //______________________________________________________________________ 00066 00079 class SerialIstreamIterator { 00080 public: 00081 typedef bistream istream_type; 00082 typedef byte value_type; 00083 typedef const byte* pointer; 00084 typedef const byte& reference; 00085 00086 SerialIstreamIterator() : stream(0), val(0) { } 00087 SerialIstreamIterator(istream_type& s) : stream(&s), val(0) { } 00088 SerialIstreamIterator& operator++() { stream->get(); return *this; } 00089 SerialIstreamIterator operator++(int) { 00090 SerialIstreamIterator i = *this; stream->get(); return i; } 00091 reference operator*() const { 00092 val = static_cast<byte>(stream->peek()); return val; } 00093 pointer operator->() const { 00094 val = static_cast<byte>(stream->peek()); return &val; } 00095 00096 private: 00097 istream_type* stream; 00098 mutable byte val; 00099 }; 00100 //____________________ 00101 00103 class SerialOstreamIterator { 00104 public: 00105 typedef bostream ostream_type; 00106 typedef void value_type; 00107 typedef void difference_type; 00108 typedef void pointer; 00109 typedef void reference; 00110 00111 SerialOstreamIterator(ostream_type& s) : stream(&s) { } 00112 SerialOstreamIterator& operator=(const byte val) { 00113 stream->put(val); 00114 return *this; 00115 } 00116 SerialOstreamIterator& operator*() { return *this; } 00117 SerialOstreamIterator& operator++() { return *this; } 00118 SerialOstreamIterator& operator++(int) { return *this; } 00119 private: 00120 ostream_type* stream; 00121 }; 00122 //______________________________________________________________________ 00123 00124 // Serializations of common data types. Stores in little-endian. 00125 //________________________________________ 00126 00130 template<class NumType, class Iterator> 00131 inline Iterator serialize1(NumType x, Iterator i) { 00132 *i = x & 0xff; ++i; 00133 return i; 00134 } 00135 template<class NumType, class ConstIterator> 00136 inline ConstIterator unserialize1(NumType& x, ConstIterator i) { 00137 x = static_cast<NumType>(*i); ++i; 00138 return i; 00139 } 00140 00141 template<class NumType, class Iterator> 00142 inline Iterator serialize2(NumType x, Iterator i) { 00143 *i = x & 0xff; ++i; 00144 *i = (x >> 8) & 0xff; ++i; 00145 return i; 00146 } 00147 template<class NumType, class ConstIterator> 00148 inline ConstIterator unserialize2(NumType& x, ConstIterator i) { 00149 x = static_cast<NumType>(*i); ++i; 00150 x |= static_cast<NumType>(*i) << 8; ++i; 00151 return i; 00152 } 00153 00154 template<class NumType, class Iterator> 00155 inline Iterator serialize4(NumType x, Iterator i) { 00156 *i = x & 0xff; ++i; 00157 *i = (x >> 8) & 0xff; ++i; 00158 *i = (x >> 16) & 0xff; ++i; 00159 *i = (x >> 24) & 0xff; ++i; 00160 return i; 00161 } 00162 template<class NumType, class ConstIterator> 00163 inline ConstIterator unserialize4(NumType& x, ConstIterator i) { 00164 x = static_cast<NumType>(*i); ++i; 00165 x |= static_cast<NumType>(*i) << 8; ++i; 00166 x |= static_cast<NumType>(*i) << 16; ++i; 00167 x |= static_cast<NumType>(*i) << 24; ++i; 00168 return i; 00169 } 00170 00171 template<class NumType, class Iterator> 00172 inline Iterator serialize6(NumType x, Iterator i) { 00173 *i = x & 0xff; ++i; 00174 *i = (x >> 8) & 0xff; ++i; 00175 *i = (x >> 16) & 0xff; ++i; 00176 *i = (x >> 24) & 0xff; ++i; 00177 *i = (x >> 32) & 0xff; ++i; 00178 *i = (x >> 40) & 0xff; ++i; 00179 return i; 00180 } 00181 template<class NumType, class ConstIterator> 00182 inline ConstIterator unserialize6(NumType& x, ConstIterator i) { 00183 x = static_cast<NumType>(*i); ++i; 00184 x |= static_cast<NumType>(*i) << 8; ++i; 00185 x |= static_cast<NumType>(*i) << 16; ++i; 00186 x |= static_cast<NumType>(*i) << 24; ++i; 00187 x |= static_cast<NumType>(*i) << 32; ++i; 00188 x |= static_cast<NumType>(*i) << 40; ++i; 00189 return i; 00190 } 00191 00192 template<class NumType, class Iterator> 00193 inline Iterator serialize8(NumType x, Iterator i) { 00194 *i = x & 0xff; ++i; 00195 *i = (x >> 8) & 0xff; ++i; 00196 *i = (x >> 16) & 0xff; ++i; 00197 *i = (x >> 24) & 0xff; ++i; 00198 *i = (x >> 32) & 0xff; ++i; 00199 *i = (x >> 40) & 0xff; ++i; 00200 *i = (x >> 48) & 0xff; ++i; 00201 *i = (x >> 56) & 0xff; ++i; 00202 return i; 00203 } 00204 template<class NumType, class ConstIterator> 00205 inline ConstIterator unserialize8(NumType& x, ConstIterator i) { 00206 x = static_cast<NumType>(*i); ++i; 00207 x |= static_cast<NumType>(*i) << 8; ++i; 00208 x |= static_cast<NumType>(*i) << 16; ++i; 00209 x |= static_cast<NumType>(*i) << 24; ++i; 00210 x |= static_cast<NumType>(*i) << 32; ++i; 00211 x |= static_cast<NumType>(*i) << 40; ++i; 00212 x |= static_cast<NumType>(*i) << 48; ++i; 00213 x |= static_cast<NumType>(*i) << 56; ++i; 00214 return i; 00215 } 00217 //______________________________________________________________________ 00218 00219 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
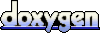