jigdo API
JigdoCache Class Reference
A list of FileParts that is "lazy": Nothing is actually read from the files passed to it until that is really necessary. More...
#include <scan.hh>
Public Types | |
typedef FilePart | value_type |
Access to the members like for a list<>. | |
typedef FilePart & | reference |
Public Member Functions | |
JigdoCache (const string &cacheFileName, size_t expiryInSeconds=60 *60 *24 *30, size_t bufLen=128 *1024, ProgressReporter &pr=noReport) | |
cacheFileName can be "" for no file cache | |
~JigdoCache () | |
The dtor will try to write cached data to the cache file. | |
template<class RecurseDir> | |
void | readFilenames (RecurseDir &rd) |
Read a list of filenames from the object and store them in the JigdoCache. | |
void | setParams (size_t blockLen, size_t md5BlockLen) |
Set the sizes of cache's blockLength and md5BlockLength parameters. | |
size_t | getBlockLen () const |
size_t | getMD5BlockLen () const |
void | setReadAmount (size_t bytes) |
Amount of data that JigdoCache will attempt to read per call to ifstream::read(), and size of buffer allocated. | |
void | deallocBuffer () |
To speed things up, JigdoCache by default keeps one file open and a read buffer allocated. | |
ProgressReporter * | getReporter () |
Return reporter supplied by JigdoCache creator. | |
void | setCheckFiles (bool check) |
Set and get whether to check if files exist in the filesystem. | |
bool | getCheckFiles () const |
size_t | size () const |
Returns number of files in cache. | |
template<class StringP, class StringL, class StringU> | |
const LocationPathSet::iterator | addLabel (const StringP &path, const StringL &label, const StringU &uri="") |
Make a label for a certain directory name known. | |
iterator | begin () |
First element of the JigdoCache. | |
iterator | end () |
NB the list auto-extends, so the value of end() may change while you iterate over a JigdoCache. | |
Friends | |
class | FilePart |
class | JigdoCache::iterator |
Classes | |
class | iterator |
Only part of the iterator functionality implemented ATM. More... | |
class | ProgressReporter |
Class allowing JigdoCache to convey information back to the creator of a JigdoCache object. More... |
Detailed Description
A list of FileParts that is "lazy": Nothing is actually read from the files passed to it until that is really necessary.JigdoCache behaves like a list<FilePart>.
A JigdoCache cannot hold zero-length files. They will be silently skipped when you try to add them.
Important note: If you decide to throw the errors handed to your errorHandler, JigdoCacheErrors can be thrown by some methods. The default cerrPrint (guess what) only outputs the error message to cerr.
Member Typedef Documentation
typedef FilePart JigdoCache::value_type |
Access to the members like for a list<>.
typedef FilePart& JigdoCache::reference |
Constructor & Destructor Documentation
JigdoCache::JigdoCache | ( | const string & | cacheFileName, | |
size_t | expiryInSeconds = 60*60*24*30 , |
|||
size_t | bufLen = 128*1024 , |
|||
ProgressReporter & | pr = noReport | |||
) | [explicit] |
cacheFileName can be "" for no file cache
References _, JigdoCache::ProgressReporter::error(), Error::message, and subst.
JigdoCache::~JigdoCache | ( | ) |
The dtor will try to write cached data to the cache file.
References _, debug, JigdoCache::ProgressReporter::error(), CacheFile::expire(), CacheFile::insert(), Error::message, Paranoid, and subst.
Member Function Documentation
void JigdoCache::readFilenames | ( | RecurseDir & | rd | ) | [inline] |
Read a list of filenames from the object and store them in the JigdoCache.
Only the file size is read during the call, Rsync/MD5 sums are calculated later, if/when needed.
References _, data, JigdoCache::ProgressReporter::error(), Status::failed(), CacheFile::findName(), RecurseDir::getName(), Error::message, and subst.
Referenced by main().
void JigdoCache::setParams | ( | size_t | blockLen, | |
size_t | md5BlockLen | |||
) |
Set the sizes of cache's blockLength and md5BlockLength parameters.
This means that the checksum returned by a FilePart's getRsyncSum() method will cover the specified size. NB: If the cache already contains files and the new parameters are not the same as the old, the start of the files will (eventually) have to be re-read to re-calculate the checksums for blockLength, and the *whole* file will have to be re-read for a changed md5BlockLength.
Referenced by main().
size_t JigdoCache::getBlockLen | ( | ) | const [inline] |
Referenced by MkTemplate::run().
size_t JigdoCache::getMD5BlockLen | ( | ) | const [inline] |
Referenced by MkTemplate::run().
void JigdoCache::setReadAmount | ( | size_t | bytes | ) | [inline] |
Amount of data that JigdoCache will attempt to read per call to ifstream::read(), and size of buffer allocated.
Minimum: 64k
void JigdoCache::deallocBuffer | ( | ) | [inline] |
To speed things up, JigdoCache by default keeps one file open and a read buffer allocated.
You can close the file and deallocate the buffer with a call to this method. They will be re-opened/re-allocated automatically if/when needed.
Referenced by MkTemplate::run().
ProgressReporter* JigdoCache::getReporter | ( | ) | [inline] |
Return reporter supplied by JigdoCache creator.
void JigdoCache::setCheckFiles | ( | bool | check | ) | [inline] |
Set and get whether to check if files exist in the filesystem.
bool JigdoCache::getCheckFiles | ( | ) | const [inline] |
size_t JigdoCache::size | ( | ) | const [inline] |
Returns number of files in cache.
const LocationPathSet::iterator JigdoCache::addLabel | ( | const StringP & | path, | |
const StringL & | label, | |||
const StringU & | uri = "" | |||
) | [inline] |
Make a label for a certain directory name known.
E.g. addLabel("pub/debian/","deb")
will cause the file pub/debianf;/dists/stable/x.deb
to appear as deb:dists/stable/x.deb
in the location list. Each of the paramters can bei either string
or const char*
. Note: If no labels are defined, a name will automatically be created later: dirA, dirB, ..., dirZ, dirAA, ...
References LocationPath::label, LocationPath::path, and LocationPath::uri.
JigdoCache::iterator JigdoCache::begin | ( | ) | [inline] |
iterator JigdoCache::end | ( | ) | [inline] |
NB the list auto-extends, so the value of end() may change while you iterate over a JigdoCache.
References JigdoCache::iterator::iterator().
Referenced by JigdoDesc::makeImage(), JigdoCache::iterator::operator++(), MkTemplate::run(), and setParams().
Friends And Related Function Documentation
friend class FilePart [friend] |
friend class JigdoCache::iterator [friend] |
The documentation for this class was generated from the following files:
Generated on Tue Sep 23 14:27:42 2008 for jigdo by
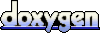