jigdo API
Last update by Admin on 2010-05-23
util/log.hh
Go to the documentation of this file.00001 /* $Id: log.hh,v 1.5 2003/08/17 15:37:07 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2003 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00052 #ifndef LOG_HH 00053 #define LOG_HH 00054 00055 #include <config.h> 00056 #include <debug.hh> 00057 #include <nocopy.hh> 00058 #include <string-utf.hh> 00059 //______________________________________________________________________ 00060 00061 #if DEBUG 00062 # define DEBUG_UNIT(_name) namespace { Logger debug(_name); } 00063 # define LOCAL_DEBUG_UNIT(_name) Logger debug(_name); 00064 # define LOCAL_DEBUG_UNIT_DECL static Logger debug; 00065 # define DEBUG_TO(_realobject) namespace { Logger& debug(_realobject); } 00066 # define LOCAL_DEBUG_TO(_realobject) Logger& debug(_realobject); 00067 #else 00068 # if HAVE_VARMACRO 00069 # define DEBUG_UNIT(_name) 00070 # define LOCAL_DEBUG_UNIT(_name) 00071 # define LOCAL_DEBUG_UNIT_DECL 00072 # define DEBUG_TO(_realobject) 00073 # define LOCAL_DEBUG_TO(_realobject) 00074 # if defined(__GNUC__) && __GNUC__ < 3 00075 # define debug(_args...) do { } while (false) 00076 # else 00077 # define debug(...) do { } while (false) 00078 # endif 00079 # else 00080 # define DEBUG_UNIT(_name) namespace { IgnoreLogger debug; } 00081 # define LOCAL_DEBUG_UNIT(_name) IgnoreLogger debug; 00082 # define LOCAL_DEBUG_UNIT_DECL IgnoreLogger debug; 00083 # define DEBUG_TO(_realobject) namespace { IgnoreLogger debug; } 00084 # define LOCAL_DEBUG_TO(_realobject) IgnoreLogger debug; 00085 /* Var-arg macros not supported, we have to define a dummy class. 00086 Disadvantage: Arguments of debug() will be evaluated even in 00087 non-debug builds. */ 00088 struct IgnoreLogger : NoCopy { 00089 struct S { template<class X> S(const X&) { } }; 00090 bool enabled() const { return false; } 00091 operator bool() const { return false; } 00092 void operator()(const char*) const { } 00093 void operator()(const char*, S) const { } 00094 void operator()(const char*, S, S) const { } 00095 void operator()(const char*, S, S, S) const { } 00096 void operator()(const char*, S, S, S, S) const { } 00097 void operator()(const char*, S, S, S, S, S) const { } 00098 void operator()(const char*, S, S, S, S, S, S) const { } 00099 void operator()(const char*, S, S, S, S, S, S, S) const { } 00100 void operator()(const char*, S, S, S, S, S, S, S, S) const { } 00101 void operator()(const char*, S, S, S, S, S, S, S, S, S) const { } 00102 }; 00103 # endif 00104 #endif 00105 //______________________________________________________________________ 00106 00109 class Logger : NoCopy { 00110 public: 00111 00112 # ifndef DOXYGEN_SKIP 00113 /* Logged strings are output via a OutputFunction* pointer. */ 00114 typedef void (OutputFunction)(const string& unitName, 00115 unsigned char unitNameLen, const char* format, int args, 00116 const Subst arg[]); 00117 # endif 00118 00119 static void defaultPut(const string& unitName, unsigned char unitNameLen, 00120 const char* format, int args, const Subst arg[]); 00122 static void setOutputFunction(OutputFunction* newOut) { output = newOut; } 00123 00127 Logger(const char* unitName, bool enabled = false); 00128 bool enabled() const { return enabledVal; } 00129 operator bool() const { return enabledVal; } 00130 const char* name() const { return unitNameVal; } 00131 00137 static bool setEnabled(const char* unitName, bool enable = true); 00138 00146 static inline Logger* enumerate(Logger* l = 0) { 00147 return (l ? l->next : list); } 00148 00149 void operator()(const char* format) const { 00150 if (enabled()) put(format, 0, 0); 00151 } 00152 void operator()(const char* format, Subst a) const { 00153 if (enabled()) put(format, 1, &a); 00154 } 00155 void operator()(const char* format, Subst a, Subst b) const { 00156 if (!enabled()) return; 00157 Subst arg[] = { a, b }; 00158 put(format, 2, arg); 00159 } 00160 void operator()(const char* format, Subst a, Subst b, Subst c) const { 00161 if (!enabled()) return; 00162 Subst arg[] = { a, b, c }; 00163 put(format, 3, arg); 00164 } 00165 void operator()(const char* format, Subst a, Subst b, Subst c, Subst d) 00166 const { 00167 if (!enabled()) return; 00168 Subst arg[] = { a, b, c, d }; 00169 put(format, 4, arg); 00170 } 00171 void operator()(const char* format, Subst a, Subst b, Subst c, Subst d, 00172 Subst e) const { 00173 if (!enabled()) return; 00174 Subst arg[] = { a, b, c, d, e }; 00175 put(format, 5, arg); 00176 } 00177 void operator()(const char* format, Subst a, Subst b, Subst c, Subst d, 00178 Subst e, Subst f) const { 00179 if (!enabled()) return; 00180 Subst arg[] = { a, b, c, d, e, f }; 00181 put(format, 6, arg); 00182 } 00183 void operator()(const char* format, Subst a, Subst b, Subst c, Subst d, 00184 Subst e, Subst f, Subst g) const { 00185 if (!enabled()) return; 00186 Subst arg[] = { a, b, c, d, e, f, g }; 00187 put(format, 7, arg); 00188 } 00189 void operator()(const char* format, Subst a, Subst b, Subst c, Subst d, 00190 Subst e, Subst f, Subst g, Subst h) const { 00191 if (!enabled()) return; 00192 Subst arg[] = { a, b, c, d, e, f, g, h }; 00193 put(format, 8, arg); 00194 } 00195 void operator()(const char* format, Subst a, Subst b, Subst c, Subst d, 00196 Subst e, Subst f, Subst g, Subst h, Subst i) const { 00197 if (!enabled()) return; 00198 Subst arg[] = { a, b, c, d, e, f, g, h, i }; 00199 put(format, 9, arg); 00200 } 00201 00207 static void scanOptions(const string& s, const char* binName); 00208 00209 private: 00210 static OutputFunction* output; 00211 void put(const char* format, int args, const Subst arg[]) const { 00212 output(unitNameVal, unitNameLen, format, args, arg); 00213 } 00214 00215 static Logger* list; // Linked list of registered units 00216 static string buf; // Temporary buffer for output string, printed at endl 00217 00218 const char* unitNameVal; 00219 unsigned char unitNameLen; 00220 bool enabledVal; // only print messages if true 00221 Logger* next; // Next in linked list, or null 00222 }; 00223 //______________________________________________________________________ 00224 00228 extern Logger msg; 00229 //______________________________________________________________________ 00230 00231 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
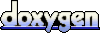