jigdo API
Last update by Admin on 2010-05-23
util/string.hh
Go to the documentation of this file.00001 /* $Id: string.hh,v 1.1.1.1 2003/07/04 22:30:12 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2001-2002 | richard@ 00004 | \/�| Richard Atterer | atterer.net 00005 � '` � 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00017 #ifndef STRING_HH 00018 #define STRING_HH 00019 00020 #include <config.h> 00021 00022 #include <string> 00023 //______________________________________________________________________ 00024 00026 string& append(string& s, double x); 00027 string& append(string& s, int x); 00028 string& append(string& s, unsigned x); 00029 string& append(string& s, long x); 00030 string& append(string& s, unsigned long x); 00031 #if HAVE_UNSIGNED_LONG_LONG 00032 string& append(string& s, unsigned long long x); 00033 #endif 00034 00036 string& append(string& s, unsigned x, int width); 00037 string& append(string& s, unsigned long x, int width); 00038 #if HAVE_UNSIGNED_LONG_LONG 00039 string& append(string& s, unsigned long long x, int width); 00040 #endif 00041 //______________________________________________________________________ 00042 00044 class Subst { 00045 public: 00046 Subst(int x) { type = INT; val.intVal = x; } 00047 Subst(unsigned x) { type = UNSIGNED; val.unsignedVal = x; } 00048 Subst(long x) { type = LONG; val.longVal = x; } 00049 Subst(unsigned long x) { type = ULONG; val.ulongVal = x; } 00050 # if HAVE_UNSIGNED_LONG_LONG 00051 Subst(unsigned long long x) { type = ULONGLONG; val.ulonglongVal = x; } 00052 # endif 00053 Subst(double x) { type = DOUBLE; val.doubleVal = x; } 00054 Subst(char x) { type = CHAR; val.charVal = x; } 00055 Subst(const char* x) { type = CHAR_P; val.charPtr = x; } 00056 Subst(const string& x) { type = STRING_P; val.stringPtr = &x; } 00057 Subst(const string* x) { type = STRING_P; val.stringPtr = x; } 00058 Subst(const void* x) { type = POINTER; val.pointerVal = x; } 00059 static string subst(const char* format, int args, const Subst arg[]); 00060 00061 private: 00062 00063 # ifndef DOXYGEN_SKIP 00064 # ifdef STRING_UTF_HH 00065 static inline void doSubst(string& result, const Subst arg[], int n, 00066 int flags); 00067 # endif 00068 # endif 00069 enum { 00070 INT, UNSIGNED, LONG, ULONG, ULONGLONG, DOUBLE, CHAR, CHAR_P, STRING_P, 00071 POINTER 00072 } type; 00073 union { 00074 int intVal; 00075 unsigned unsignedVal; 00076 long longVal; 00077 unsigned long ulongVal; 00078 # if HAVE_UNSIGNED_LONG_LONG 00079 unsigned long long ulonglongVal; 00080 # endif 00081 double doubleVal; 00082 char charVal; 00083 const char* charPtr; 00084 const string* stringPtr; 00085 const void* pointerVal; 00086 } val; 00087 }; 00088 //______________________________________________________________________ 00089 00090 /* Example: 00091 cout << subst("file `%1' not found: %2", 00092 string("foo"), strerror(errno)); */ 00093 00094 inline string subst(const char* format, Subst a) { 00095 return Subst::subst(format, 1, &a); 00096 } 00097 inline string subst(const char* format, Subst a, Subst b) { 00098 Subst arg[] = { a, b }; 00099 return Subst::subst(format, 2, arg); 00100 } 00101 inline string subst(const char* format, Subst a, Subst b, Subst c) { 00102 Subst arg[] = { a, b, c }; 00103 return Subst::subst(format, 3, arg); 00104 } 00105 inline string subst(const char* format, Subst a, Subst b, Subst c, Subst d) { 00106 Subst arg[] = { a, b, c, d }; 00107 return Subst::subst(format, 4, arg); 00108 } 00109 inline string subst(const char* format, Subst a, Subst b, Subst c, Subst d, 00110 Subst e) { 00111 Subst arg[] = { a, b, c, d, e }; 00112 return Subst::subst(format, 5, arg); 00113 } 00114 inline string subst(const char* format, Subst a, Subst b, Subst c, Subst d, 00115 Subst e, Subst f) { 00116 Subst arg[] = { a, b, c, d, e, f }; 00117 return Subst::subst(format, 6, arg); 00118 } 00119 inline string subst(const char* format, Subst a, Subst b, Subst c, Subst d, 00120 Subst e, Subst f, Subst g) { 00121 Subst arg[] = { a, b, c, d, e, f, g }; 00122 return Subst::subst(format, 7, arg); 00123 } 00124 inline string subst(const char* format, Subst a, Subst b, Subst c, Subst d, 00125 Subst e, Subst f, Subst g, Subst h) { 00126 Subst arg[] = { a, b, c, d, e, f, g, h }; 00127 return Subst::subst(format, 8, arg); 00128 } 00129 inline string subst(const char* format, Subst a, Subst b, Subst c, Subst d, 00130 Subst e, Subst f, Subst g, Subst h, Subst i) { 00131 Subst arg[] = { a, b, c, d, e, f, g, h, i }; 00132 return Subst::subst(format, 9, arg); 00133 } 00134 00135 #endif
Generated on Tue Sep 23 14:27:42 2008 for jigdo by
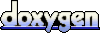