jigdo API
GtkSingleUrl Class Reference
This class is two things at once:. More...
#include <gtk-single-url.hh>
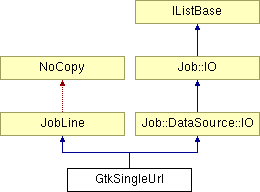
Public Types | |
typedef void(GtkSingleUrl::* | TickHandler )() |
Public Member Functions | |
GtkSingleUrl (const string &uriStr, const string &destFile) | |
Create a new GtkSingleUrl, and also create an internal Job::SingleUrl to do the actual download. | |
GtkSingleUrl (const string &uriStr, const string &destDesc, Job::DataSource *download) | |
Create a new GtkSingleUrl, but use it with an already existing Job::DataSource. | |
virtual | ~GtkSingleUrl () |
virtual bool | run () |
Start/restart this job. | |
virtual void | selectRow () |
Called when the JobLine's line is selected in the list. | |
virtual bool | paused () const |
Is the job currently paused? | |
virtual void | pause () |
Pause the job. | |
virtual void | cont () |
Continue executing the job. | |
virtual void | stop () |
Stop executing the job. | |
virtual void | percentDone (uint64 *cur, uint64 *total) |
When called, must fill in current nr of bytes downloaded and total nr of bytes. | |
void | callRegularly (TickHandler handler) |
void | callRegularlyLater (const int milliSec, TickHandler handler) |
void | on_startButton_clicked () |
void | on_pauseButton_clicked () |
void | on_stopButton_clicked () |
void | on_restartButton_clicked () |
void | on_closeButton_clicked () |
void | childIsFinished () |
Cause this child download to remove itself from the JobList after CHILD_FINISHED_DELAY. | |
Static Public Attributes | |
static const int | CHILD_FINISHED_DELAY = 10000 |
Only in child mode, delay (millisec) between the MakeImageDl telling us that it has deleted its child and the moment we delete the corresponding line from the JobList. |
Detailed Description
This class is two things at once:.1) Normal GtkSingleUrl mode: The frontend for a single-file download. It is a JobLine, so can be paused etc. like all JobLines. It registers as a Job::SingleUrl::IO with the Job::SingleUrl _that_it_owns_, to be notified whenever the job has something to say.
2) GtkDataSource-which-just-isnt-called-like-that, aka "child mode": The frontend for a Job::DataSource object. This DataSource object is _not_ owned by the GtkSingleUrl object. This is used if a MakeImageDl starts new child downloads.
The two modes share so much code that IMHO doing two classes would not be better.
Member Typedef Documentation
typedef void(GtkSingleUrl::* GtkSingleUrl::TickHandler)() |
Reimplemented from JobLine.
Constructor & Destructor Documentation
GtkSingleUrl::GtkSingleUrl | ( | const string & | uriStr, | |
const string & | destFile | |||
) |
Create a new GtkSingleUrl, and also create an internal Job::SingleUrl to do the actual download.
Delete the internal SingleURl from ~GtkSingleUrl().
References debug.
GtkSingleUrl::GtkSingleUrl | ( | const string & | uriStr, | |
const string & | destDesc, | |||
Job::DataSource * | download | |||
) |
Create a new GtkSingleUrl, but use it with an already existing Job::DataSource.
The Job::DataSource will not be owned by the GtkSingleUrl and will not be deleted by its dtor. This mode is used to create "child downloads" during .jigdo processing: The IO object registered with the supplied Job::DataSource is set up to do with the data what it wants, and then to pass the call on to this GtkSingleUrl (more accurately, the methods that this GtkSingleUrl inherits from Job::DataSource::IO). See also GtkMakeImage::makeImageDl_new().
- Parameters:
-
uriStr URL destDesc A descriptive string like "/foo/bar/image, offset 3453", NOT a filename! Supplied for information only, to be displayed to the user. download The download we are attached to
References debug.
GtkSingleUrl::~GtkSingleUrl | ( | ) | [virtual] |
References callRegularly(), debug, JobLine::jobList(), Paranoid, setNotebookPage(), and GUI::window.
Member Function Documentation
bool GtkSingleUrl::run | ( | ) | [virtual] |
Start/restart this job.
When called, must also create GUI elements for this Job in row number row() of GtkTreeView jobList()->view(). Is called after Job is added to the list, and when it is restarted (if at all). Child classes should provide an implementation.
- Returns:
- SUCCESS if everything OK, FAILURE if this object has deleted itself.
Implements JobLine.
References _, JobList::COLUMN_OBJECT, JobList::COLUMN_STATUS, MessageBox::ERROR, JobLine::jobList(), MessageBox::OK, JobLine::row(), MessageBox::Ref::set(), MessageBox::show(), and subst.
Referenced by JobLine::create().
void GtkSingleUrl::selectRow | ( | ) | [virtual] |
Called when the JobLine's line is selected in the list.
Implements JobLine.
References JobLine::jobList(), setNotebookPage(), JobList::setWindowOwner(), and GUI::window.
bool GtkSingleUrl::paused | ( | ) | const [virtual] |
Is the job currently paused?
Implements JobLine.
Referenced by cont(), on_pauseButton_clicked(), on_restartButton_clicked(), on_startButton_clicked(), and pause().
void GtkSingleUrl::pause | ( | ) | [virtual] |
Pause the job.
Implements JobLine.
References _, Job::DataSource::pause(), and paused().
Referenced by on_pauseButton_clicked().
void GtkSingleUrl::cont | ( | ) | [virtual] |
Continue executing the job.
Implements JobLine.
References Job::DataSource::cont(), and paused().
Referenced by on_startButton_clicked().
void GtkSingleUrl::stop | ( | ) | [virtual] |
Stop executing the job.
Implements JobLine.
References _, Job::SingleUrl::cont(), Progress::currentSize(), debug, Paranoid, Job::SingleUrl::progress(), Job::SingleUrl::setDestination(), and Job::SingleUrl::stop().
Referenced by on_stopButton_clicked().
void GtkSingleUrl::percentDone | ( | uint64 * | cur, | |
uint64 * | total | |||
) | [virtual] |
When called, must fill in current nr of bytes downloaded and total nr of bytes.
Implements JobLine.
References Progress::currentSize(), Progress::dataSize(), and Job::DataSource::progress().
void GtkSingleUrl::callRegularly | ( | TickHandler | handler | ) | [inline] |
void GtkSingleUrl::callRegularlyLater | ( | const int | milliSec, | |
TickHandler | handler | |||
) | [inline] |
void GtkSingleUrl::on_startButton_clicked | ( | ) |
References _, MessageBox::addButton(), MessageBox::addStockButton(), Assert, cont(), Progress::currentSize(), MessageBox::ERROR, MessageBox::NONE, MessageBox::OK, MessageBox::onResponse(), Paranoid, paused(), Job::DataSource::progress(), MessageBox::Ref::set(), MessageBox::show(), size, subst, MessageBox::WARNING, and GUI::window.
Referenced by on_download_startButton_clicked().
void GtkSingleUrl::on_pauseButton_clicked | ( | ) | [inline] |
void GtkSingleUrl::on_stopButton_clicked | ( | ) | [inline] |
void GtkSingleUrl::on_restartButton_clicked | ( | ) |
References _, MessageBox::addButton(), MessageBox::addStockButton(), Progress::currentSize(), debug, MessageBox::NONE, MessageBox::onResponse(), paused(), Job::DataSource::progress(), MessageBox::Ref::set(), MessageBox::show(), Progress::speed(), and MessageBox::WARNING.
Referenced by on_download_restartButton_clicked().
void GtkSingleUrl::on_closeButton_clicked | ( | ) |
void GtkSingleUrl::childIsFinished | ( | ) | [inline] |
Cause this child download to remove itself from the JobList after CHILD_FINISHED_DELAY.
References callRegularlyLater(), and CHILD_FINISHED_DELAY.
Member Data Documentation
const int GtkSingleUrl::CHILD_FINISHED_DELAY = 10000 [static] |
Only in child mode, delay (millisec) between the MakeImageDl telling us that it has deleted its child and the moment we delete the corresponding line from the JobList.
The delay allows the user to read the "finished" message.
Referenced by childIsFinished().
The documentation for this class was generated from the following files:
Generated on Tue Sep 23 14:27:42 2008 for jigdo by
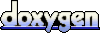