jigdo API
Last update by Admin on 2010-05-23
gtk/jobline.hh
Go to the documentation of this file.00001 /* $Id: jobline.hh,v 1.2 2003/08/17 15:37:07 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2003 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00016 #ifndef JOBLINE_HH 00017 #define JOBLINE_HH 00018 00019 #include <config.h> 00020 #include <gtk/gtk.h> 00021 00022 #include <nocopy.hh> 00023 #include <joblist.hh> 00024 //______________________________________________________________________ 00025 00028 class JobLine : NoCopy { 00029 friend class JobList; // Overwrites jobVec and rowVal on insert() 00030 public: 00031 inline JobLine(); 00032 00036 virtual ~JobLine(); 00037 00044 virtual bool run() = 0; 00045 00047 virtual void selectRow() = 0; 00048 00050 virtual bool paused() const = 0; 00052 virtual void pause() = 0; 00054 virtual void cont() = 0; 00056 virtual void stop() = 0; 00057 00060 virtual void percentDone(uint64* cur, uint64* total) = 0; 00061 00069 static void create(const char* uri, const char* dest); 00070 00071 protected: 00072 typedef void (JobLine::*TickHandler)(); 00074 inline JobList* jobList() const; 00079 inline GtkTreeIter* row(); 00080 00089 inline void callRegularly(TickHandler handler); 00091 TickHandler getHandler() const { return tick; } 00093 bool needTicks() const { return tick != 0; } 00097 inline void callRegularlyLater(const int milliSec, TickHandler handler); 00098 00099 private: 00100 void waitTick(); 00101 int waitCountdown; 00102 TickHandler waitDestination; 00103 00104 JobList* jobVec; 00105 TickHandler tick; 00106 /* A reference to the row that this JobLine object is stored in. 00107 GtkTreeModel doc sez: GtkTreeStore and GtkListStore "guarantee that an 00108 iterator is valid for as long as the node it refers to is valid" */ 00109 GtkTreeIter rowVal; 00110 }; 00111 00112 //====================================================================== 00113 00114 JobLine::JobLine() : jobVec(0), tick(0) { } 00115 JobList* JobLine::jobList() const { return jobVec; } 00116 GtkTreeIter* JobLine::row() { return &rowVal; } 00117 //________________________________________ 00118 00119 void JobLine::callRegularly(TickHandler handler) { 00120 if (!needTicks() && handler != 0) 00121 jobList()->registerTicks(); // no previous handler, now handler 00122 else if (needTicks() && handler == 0) 00123 jobList()->unregisterTicks(); // previous handler present, now none 00124 tick = handler; 00125 } 00126 00127 void JobLine::callRegularlyLater(const int milliSec, TickHandler handler) { 00128 waitCountdown = milliSec / JobList::TICK_INTERVAL; 00129 waitDestination = handler; 00130 callRegularly(&JobLine::waitTick); 00131 } 00132 00133 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
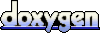