jigdo API
Last update by Admin on 2010-05-23
util/progress.hh
Go to the documentation of this file.00001 /* $Id: progress.hh,v 1.7 2003/08/15 11:38:30 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2003 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00016 #ifndef PROGRESS_HH 00017 #define PROGRESS_HH 00018 00019 #include <config.h> 00020 00021 #include <iosfwd> 00022 #include <string> 00023 #include <glib.h> 00024 00025 #include <progress.fh> 00026 //______________________________________________________________________ 00027 00030 class Progress { 00031 public: 00032 inline Progress(); 00033 inline ~Progress(); 00034 00036 inline void setCurrentSize(uint64 x); 00038 inline uint64 currentSize() const; 00040 inline void setDataSize(uint64 x); 00042 inline uint64 dataSize() const; 00043 00051 void tick(const GTimeVal& now, int millisecs); 00059 void setAutoTick(bool enable); 00061 inline bool autoTick(); 00064 int speed(const GTimeVal& now) const; 00067 int timeLeft(const GTimeVal& now) const; 00068 00070 static void appendSize(string* s, uint64 size); 00072 static void appendSizes(string* s, uint64 size, uint64 total); 00075 void appendProgress(string* s) const; 00078 void appendSpeed(string* s, int speed, int timeLeft) const; 00079 00087 void reset(); 00088 00089 static const int SPEED_TICK_INTERVAL = 3000; 00090 00091 ostream& put(ostream& s) const; 00092 00093 private: 00094 // Bytes downloaded so far 00095 uint64 currentSizeVal; 00096 // Total bytes, or 0 for don't know 00097 uint64 dataSizeVal; 00098 00099 /* Estimation of download speed for "x kBytes per sec" display: Basic idea 00100 is to calculate the average download rate for the last 30 secs 00101 (SPEED_TICK_INTERVAL/1000*SPEED_SLOTS) all the time. To do this, we need 00102 to take note at regular intervals (SPEED_TICK_INTERVAL) of how much 00103 we've downloaded so far, and store the value for later use in one of 00104 SPEED_SLOTS slots. The slots are a "ring buffer" - the oldest value is 00105 always overwritten by the newest. */ 00106 static const int SPEED_SLOTS = 10; 00107 // If speed grows by >=x% from newer slot to older, ignores older slots 00108 static const unsigned SPEED_MAX_GROW = 130; 00109 static const unsigned SPEED_MIN_SHRINK = 70; 00110 uint64 slotSizeVal[SPEED_SLOTS]; // Value of currentSizeVal at slot start 00111 GTimeVal slotStart[SPEED_SLOTS]; // Timestamp of start of slot 00112 int currSlot; // Rotates through 0..SPEED_SLOTS-1 00113 int calcSlot; // Index of slot which is used for speed calculation 00114 int currSlotLeft; // Millisecs before a new slot is started 00115 /* Secs that timeLeft() must have *increased* by before the new 00116 value is reported. A *drop* of timeLeft() values is reported 00117 immediately. */ 00118 static const int TIME_LEFT_GROW_THRESHOLD = 5; 00119 mutable int prevTimeLeft; 00120 00121 /* Prev/next in list of autotick objects, or either one null if at 00122 start/end of list, or both null if not in list, or both null if only 00123 object in list. Yeah, keep it nice and simple! */ 00124 Progress* prev; 00125 Progress* next; 00126 // Callback function which calls tick() on the objects 00127 static gboolean autoTickCallback(gpointer); 00128 // First object in list of autotick objects, or null if list empty 00129 static Progress* autoTickList; 00130 static int autoTickId; // ID of glib event source for autoTickCallback 00131 }; 00132 00133 inline ostream& operator<<(ostream& s, const Progress& r); 00134 //______________________________________________________________________ 00135 00136 Progress::Progress() 00137 : currentSizeVal(0), dataSizeVal(0), prev(0), next(0) { reset(); } 00138 Progress::~Progress() { setAutoTick(false); } 00139 void Progress::setCurrentSize(uint64 x) { currentSizeVal = x; } 00140 uint64 Progress::currentSize() const { return currentSizeVal; } 00141 void Progress::setDataSize(uint64 x) { dataSizeVal = x; } 00142 uint64 Progress::dataSize() const { return dataSizeVal; } 00143 ostream& operator<<(ostream& s, const Progress& r) { return r.put(s); } 00144 bool Progress::autoTick() { 00145 return prev != 0 || next != 0 || autoTickList == this; 00146 } 00147 00148 #endif
Generated on Tue Sep 23 14:27:42 2008 for jigdo by
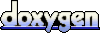