jigdo API
Last update by Admin on 2010-05-23
gtk/gtk-single-url.hh
Go to the documentation of this file.00001 /* $Id: gtk-single-url.hh,v 1.10 2003/08/30 17:43:42 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2003 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00016 #ifndef GTK_SINGLE_URL_HH 00017 #define GTK_SINGLE_URL_HH 00018 00019 #include <config.h> 00020 00021 #include <sys/types.h> 00022 #include <sys/stat.h> 00023 #include <unistd-jigdo.h> 00024 00025 #include <bstream-counted.hh> 00026 #include <jobline.hh> 00027 #include <messagebox.hh> 00028 #include <single-url.hh> 00029 //______________________________________________________________________ 00030 00045 class GtkSingleUrl : public JobLine, public Job::DataSource::IO { 00046 public: 00051 static const int CHILD_FINISHED_DELAY = 10000; 00052 00056 GtkSingleUrl(const string& uriStr, const string& destFile); 00071 GtkSingleUrl(const string& uriStr, const string& destDesc, 00072 Job::DataSource* download); 00073 virtual ~GtkSingleUrl(); 00074 00075 // Virtual methods from JobLine 00076 virtual bool run(); 00077 virtual void selectRow(); 00078 virtual bool paused() const; 00079 virtual void pause(); 00080 virtual void cont(); 00081 virtual void stop(); 00082 virtual void percentDone(uint64* cur, uint64* total); 00083 00084 typedef void (GtkSingleUrl::*TickHandler)(); 00085 inline void callRegularly(TickHandler handler); 00086 inline void callRegularlyLater(const int milliSec, TickHandler handler); 00087 00088 // Called from gui.cc 00089 void on_startButton_clicked(); 00090 inline void on_pauseButton_clicked(); 00091 inline void on_stopButton_clicked(); 00092 void on_restartButton_clicked(); 00093 void on_closeButton_clicked(); 00094 00097 inline void childIsFinished(); 00098 00099 private: 00100 enum State { 00101 CREATED, RUNNING, PAUSED, STOPPED, ERROR, SUCCEEDED 00102 }; 00103 00104 // From Job::DataSource:IO 00105 virtual void job_deleted(); 00106 virtual void job_succeeded(); 00107 virtual void job_failed(const string& message); 00108 virtual void job_message(const string& message); 00109 virtual void dataSource_dataSize(uint64 n); 00110 virtual void dataSource_data(const byte* data, unsigned size, 00111 uint64 currentSize); 00112 00113 // Helper methods 00114 /* Registered to be called for each tick by run(), updates the "% done" 00115 progress info. */ 00116 void showProgress(); 00117 // Update info in main window 00118 void updateWindow(); 00119 void resumeAsk(struct stat* fileInfo); // Ask user "resume/overwrite?" 00120 static void resumeResponse(GtkDialog*, int r, gpointer data); 00121 void openOutputAndRun(/*bool pragmaNoCache = false*/); // Allocate download job 00122 void openOutputAndResume(); // Alloc job and read resume data from file 00123 void updateTreeView(); // Update our line in GtkTreeView 00124 void failedPermanently(string* message); 00125 void startResume(); 00126 // Reload with "Pragma: no-cache" header, discards previously fetched data 00127 void restart(); 00130 void deleteThis(); 00131 // Only ask user if discarded data not worth more seconds than this 00132 static const int RESTART_WARNING_THRESHOLD = 30; 00133 static void afterStartButtonClickedResponse(GtkDialog*, int r, gpointer); 00134 static void afterCloseButtonClickedResponse(GtkDialog*, int r, gpointer); 00135 static void afterRestartButtonClickedResponse(GtkDialog*, int r, gpointer); 00136 00137 /* true if the object was created using the second ctor, i.e. with a 00138 pre-created Job::DataSource object. */ 00139 bool childMode; 00140 00141 string uri; // Source URI 00142 string dest; // Destination filename 00143 string progress, status; // Lines to display in main window 00144 string treeViewStatus; // Status section in the list of jobs 00145 SmartPtr<BfstreamCounted> destStream; 00146 00147 MessageBox::Ref messageBox; 00148 // If !childMode, the next two point to the same object! 00149 Job::DataSource* job; // Pointer to SingleUrl or to DataSource object 00150 Job::SingleUrl* singleUrl; // Pointer to SingleUrl object, or null 00151 GTimeVal pauseStart; // timestamp of last download pause, or uninitialized 00152 00153 State state; 00154 }; 00155 //______________________________________________________________________ 00156 00157 /* The static_cast from GtkSingleUrl::* to JobLine::* (i.e. member fnc of 00158 base class) is OK because we know for certain that the handler will only 00159 be invoked on SingleUrl objects. */ 00160 void GtkSingleUrl::callRegularly(TickHandler handler) { 00161 JobLine::callRegularly(static_cast<JobLine::TickHandler>(handler)); 00162 } 00163 void GtkSingleUrl::callRegularlyLater(const int milliSec, 00164 TickHandler handler) { 00165 JobLine::callRegularlyLater(milliSec, 00166 static_cast<JobLine::TickHandler>(handler)); 00167 } 00168 //________________________________________ 00169 00170 void GtkSingleUrl::on_pauseButton_clicked() { 00171 if (paused()) return; 00172 g_get_current_time(&pauseStart); 00173 pause(); 00174 gtk_label_set_text(GTK_LABEL(GUI::window.download_buttonInfo), ""); 00175 } 00176 void GtkSingleUrl::on_stopButton_clicked() { 00177 stop(); 00178 gtk_label_set_text(GTK_LABEL(GUI::window.download_buttonInfo), ""); 00179 } 00180 //________________________________________ 00181 00182 void GtkSingleUrl::childIsFinished() { 00183 if (state == SUCCEEDED) 00184 callRegularlyLater(CHILD_FINISHED_DELAY, &GtkSingleUrl::deleteThis); 00185 } 00186 00187 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
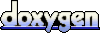