jigdo API
Last update by Admin on 2010-05-23
gtk/messagebox.hh
Go to the documentation of this file.00001 /* $Id: messagebox.hh,v 1.8 2003/06/08 22:35:23 richard Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2001-2002 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00016 #ifndef GTK_MESSAGEBOX_HH 00017 #define GTK_MESSAGEBOX_HH 00018 00019 #include <config.h> 00020 00021 #include <string> 00022 #include <gtk/gtk.h> 00023 00024 #include <debug.hh> 00025 #include <nocopy.hh> 00026 00027 #ifdef MessageBox 00028 # undef MessageBox // fsck Windows!! 00029 #endif 00030 #ifdef ERROR 00031 # undef ERROR 00032 #endif 00033 //______________________________________________________________________ 00034 00037 class MessageBox : NoCopy { 00038 public: 00039 00040 class Ref; 00041 friend class Ref; 00042 00043 enum { 00044 NONE = 0, 00045 HELP = 1 << 0, 00046 OK = 1 << 1, 00047 CLOSE = 1 << 2, 00048 CANCEL = 1 << 3 00049 }; 00050 00051 static const char* const MESSAGE; 00052 static const char* const INFO; 00053 static const char* const WARNING; 00054 static const char* const QUESTION; 00055 static const char* const ERROR; 00056 00076 inline MessageBox(const char* type, int buttons, const char* heading, 00077 const char* message = 0); 00079 inline MessageBox(const char* type, int buttons, const string& heading, 00080 const char* message); 00082 inline MessageBox(const char* type, int buttons, const char* heading, 00083 const string& message); 00085 inline MessageBox(const char* type, int buttons, const string& heading); 00087 inline MessageBox(const char* type, int buttons, const string& heading, 00088 const string& message); 00089 00090 ~MessageBox(); 00091 00098 inline MessageBox* show(); 00100 MessageBox* show_noAutoClose(); 00101 00104 GtkWidget* addButton(const char* buttonText, int response); 00105 inline GtkWidget* addButton(const char* buttonText, 00106 GtkResponseType response); 00112 GtkWidget* addStockButton(const char* buttonType, int response); 00113 inline GtkWidget* addStockButton(const char* buttonType, 00114 GtkResponseType response); 00115 00126 typedef void (*ResponseHandler)(GtkDialog*, int, gpointer); 00133 inline MessageBox* onResponse(ResponseHandler handler, gpointer data); 00134 00135 private: 00136 void init(const char* type, int buttons, const char* heading, 00137 const char* message); 00138 00139 static void destroyHandler(GtkDialog*, MessageBox* m); 00140 static void autocloseHandler(GtkDialog*, int, MessageBox* m); 00141 static void closeHandler(GtkDialog*, MessageBox* m); 00142 00143 GtkWidget* dialog; 00144 // The button to simulate a click on if Escape is pressed, or null 00145 GtkWidget* escapeButton; 00146 Ref* ref; 00147 string label; 00148 }; 00149 //______________________________________________________________________ 00150 00151 00157 class MessageBox::Ref { 00158 friend class MessageBox; 00159 public: 00160 Ref() : messageBox(0) { } 00161 inline ~Ref(); 00162 inline void set(MessageBox* m); 00163 MessageBox* get() const { return messageBox; } 00164 private: 00165 // Don't copy 00166 inline MessageBox& operator=(const MessageBox&); 00167 00168 MessageBox* messageBox; 00169 }; 00170 //______________________________________________________________________ 00171 00172 MessageBox::MessageBox(const char* type, int buttons, const char* heading, 00173 const char* message) { init(type, buttons, heading, message); } 00174 MessageBox::MessageBox(const char* type, int buttons, const string& heading, 00175 const char* message) { init(type, buttons, heading.c_str(), message); } 00176 MessageBox::MessageBox(const char* type, int buttons, const char* heading, 00177 const string& message) { init(type, buttons, heading, message.c_str()); } 00178 MessageBox::MessageBox(const char* type, int buttons, 00179 const string& heading) { init(type, buttons, heading.c_str(), 0); } 00180 MessageBox::MessageBox(const char* type, int buttons, const string& heading, 00181 const string& message) { 00182 init(type, buttons, heading.c_str(), message.c_str()); 00183 } 00184 00185 GtkWidget* MessageBox::addButton(const char* buttonText, 00186 GtkResponseType response) { 00187 return addButton(buttonText, static_cast<int>(response)); 00188 } 00189 00190 GtkWidget* MessageBox::addStockButton(const char* buttonType, 00191 GtkResponseType response) { 00192 return addStockButton(buttonType, static_cast<int>(response)); 00193 } 00194 00195 MessageBox* MessageBox::onResponse(ResponseHandler handler, gpointer data) { 00196 g_signal_connect(G_OBJECT(dialog), "response", G_CALLBACK(handler), data); 00197 return this; 00198 } 00199 00200 MessageBox* MessageBox::show() { 00201 g_signal_connect(G_OBJECT(dialog), "response", 00202 G_CALLBACK(&autocloseHandler), (gpointer)this); 00203 return show_noAutoClose(); 00204 } 00205 00206 MessageBox::Ref::~Ref() { 00207 set(0); 00208 } 00209 00210 void MessageBox::Ref::set(MessageBox* m) { 00211 if (messageBox == m) return; 00212 if (messageBox != 0) { 00213 messageBox->ref = 0; 00214 delete messageBox; 00215 } 00216 if (m != 0) { 00217 Assert(m->ref == 0); 00218 m->ref = this; 00219 } 00220 messageBox = m; 00221 } 00222 00223 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
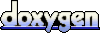