jigdo API
Last update by Admin on 2010-05-23
mktemplate.hh
Go to the documentation of this file.00001 /* $Id: mktemplate.hh,v 1.1.1.1 2003/07/04 22:29:31 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2000-2002 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00016 #ifndef MKTEMPLATE_HH 00017 #define MKTEMPLATE_HH 00018 00019 #ifndef INLINE 00020 # ifdef NOINLINE 00021 # define INLINE 00022 # else 00023 # define INLINE inline 00024 # endif 00025 #endif 00026 00027 #include <config.h> 00028 00029 #include <iostream> 00030 #include <string> 00031 #include <set> 00032 #include <vector> 00033 #include <iosfwd> 00034 00035 #include <bstream.hh> 00036 #include <compat.hh> 00037 #include <configfile.hh> 00038 #include <debug.hh> 00039 #include <jigdoconfig.fh> 00040 #include <log.hh> 00041 #include <md5sum.hh> 00042 #include <rsyncsum.hh> 00043 #include <scan.fh> 00044 #include <zstream.fh> 00045 //______________________________________________________________________ 00046 00053 class MkTemplate { 00054 public: 00055 class ProgressReporter; 00056 00074 MkTemplate(JigdoCache* jcache, bistream* imageStream, 00075 JigdoConfig* jigdoInfo, bostream* templateStream, 00076 ProgressReporter& pr = noReport, int zipQuality = 9, 00077 size_t readAmnt = 128U*1024, bool addImage = true, 00078 bool addServers = true, bool useBzip2 = false); 00079 inline ~MkTemplate(); 00080 00082 inline void setMatchExec(const string& me); 00083 00087 inline void setGreedyMatching(bool x) { greedyMatching = x; } 00088 inline bool getGreedyMatching() const { return greedyMatching; } 00089 00102 bool run(const string& imageLeafName = "image", 00103 const string& templLeafName = "template"); 00104 00106 static ProgressReporter noReport; 00107 00108 private: 00109 // Values for codes in the template data's DESC section 00110 static const byte IMAGE_INFO = 1, UNMATCHED_DATA = 2, MATCHED_FILE = 3; 00111 static const size_t REPORT_INTERVAL = 256U*1024; 00112 /* If the queue of matches runs full, we enter a special mode where new 00113 matches are only added to the queue if their start offset is a multiple 00114 of this assumed sector size. This is a heuristics - matches are more 00115 likely to start at "even" offsets. Because the actual sector size of the 00116 input image is not known, we'll prefer 00117 SECTOR_LENGTH-aligned matches to matches at odd offsets, 00118 2*SECTOR_LENGTH-aligned ones to SECTOR_LENGTH-aligned ones, 00119 4*SECTOR_LENGTH-aligned ones to 2*SECTOR_LENGTH-aligned ones etc. 00120 Initial value for sectorLength. */ 00121 static const unsigned INITIAL_SECTOR_LENGTH = 512; 00122 static const unsigned MAX_SECTOR_LENGTH = 65536; 00123 00124 /* debug(...) may be defined as a CPP macro. Luckily, that won't affect 00125 this occurance of the word. */ 00126 static Logger debug; 00127 00128 // Disallow assignment; op is never defined 00129 inline MkTemplate& operator=(const MkTemplate&); 00130 00131 // Various helper classes and functions for run() 00132 class Desc; 00133 class PartialMatch; 00134 class PartialMatchQueue; 00135 friend class PartialMatchQueue; 00136 void prepareJigdo(); 00137 void finalizeJigdo(const string& imageLeafName, 00138 const string& templLeafName, const MD5Sum& templMd5Sum); 00139 INLINE bool scanFiles(size_t blockLength, uint32 blockMask, 00140 size_t md5BlockLength); 00141 INLINE bool scanImage(byte* buf, size_t bufferLength, size_t blockLength, 00142 uint32 blockMask, size_t md5BlockLength, MD5Sum&); 00143 static INLINE void insertInTodo(PartialMatchQueue& matches, 00144 PartialMatch* x); 00145 void checkRsyncSumMatch2(const size_t blockLen, const size_t back, 00146 const size_t md5BlockLength, uint64& nextEvent, FilePart* file); 00147 INLINE void checkRsyncSumMatch(const RsyncSum64& sum, 00148 const uint32& bitMask, const size_t blockLen, const size_t back, 00149 const size_t md5BlockLength, uint64& nextEvent); 00150 INLINE bool checkMD5Match(byte* const buf, 00151 const size_t bufferLength, const size_t data, 00152 const size_t md5BlockLength, uint64& nextEvent, 00153 const size_t stillBuffered, Desc& desc); 00154 INLINE bool checkMD5Match_mismatch(const size_t stillBuffered, 00155 PartialMatch* x, Desc& desc); 00156 INLINE bool unmatchedAtEnd(byte* const buf, const size_t bufferLength, 00157 const size_t data, Desc& desc); 00158 bool rereadUnmatched(FilePart* file, uint64 count); 00159 INLINE void scanImage_mainLoop_fastForward(uint64 nextEvent, 00160 RsyncSum64* rsum, byte* buf, size_t* data, size_t* n, size_t* rsumBack, 00161 size_t bufferLength, size_t blockLength, uint32 blockMask, 00162 size_t md5BlockLength); 00163 INLINE bool matchExecCommands(PartialMatch* x); 00164 00165 inline void debugRangeInfo(uint64 start, uint64 end, const char* msg, 00166 const PartialMatch* x = 0); 00167 void printRangeInfo(uint64 start, uint64 end, const char* msg, 00168 const PartialMatch* x = 0); 00169 void debugRangeFailed(); 00170 00171 uint64 fileSizeTotal; // Accumulated lengths of all the files 00172 size_t fileCount; // Total number of files added 00173 00174 // Look up list of FileParts by hash of RsyncSum 00175 typedef vector<vector<FilePart*> > FileVec; 00176 FileVec block; 00177 00178 // Nr of bytes to read in one go, as specified by caller of MkTemplate() 00179 size_t readAmount; 00180 00181 /* The area delimited by unmatchedStart (incl) and off (excl) has "not been 00182 dealt with", either by writing it to zip, or by a match with the first 00183 MD5 block of an input file. Once a partial match of a file has been 00184 detected, unmatchedStart "gets stuck" at the start offset of this file 00185 within the image. */ 00186 uint64 off; // Current absolute offset in image 00187 uint64 unmatchedStart; 00188 00189 bool greedyMatching; 00190 00191 JigdoCache* cache; 00192 bistream* image; 00193 bostream* templ; 00194 Zobstream* zip; // Compressing stream for template data output 00195 00196 int zipQual; // 0..9, passed to zlib 00197 ProgressReporter& reporter; 00198 PartialMatchQueue* matches; // queue of partially matched files 00199 unsigned sectorLength; 00200 //____________________ 00201 00202 JigdoConfig* jigdo; 00203 struct PartLine; 00204 struct PartIndex; 00205 set<PartLine> jigdoParts; // lines of [Parts] section 00206 vector<FilePart*> matchedParts; // New parts to add to [Parts] at end 00207 // true => add a [Image/Servers] section to the output .jigdo file 00208 bool addImageSection; 00209 bool addServersSection; 00210 bool useBzLib; 00211 string matchExec; 00212 //____________________ 00213 00214 // For debugging of the template creation 00215 uint64 oldAreaEnd; 00216 }; 00217 00218 #include <partialmatch.hh> 00219 //______________________________________________________________________ 00220 00226 class MkTemplate::ProgressReporter { 00227 public: 00228 virtual ~ProgressReporter() { } 00230 virtual void error(const string& message); 00233 virtual void scanningImage(uint64 offset); 00234 // Error while reading the image file - aborting immediately 00235 // virtual void abortingScan(); 00240 virtual void matchFound(const FilePart* file, uint64 offInImage); 00243 virtual void finished(uint64 imageSize); 00244 }; 00245 //______________________________________________________________________ 00246 00249 struct MkTemplate::PartLine { 00250 string text; 00251 size_t split; // Offset of first char after "foo" 00252 bool operator<(const PartLine& x) const { 00253 int cmp = text.compare(x.text); 00254 return cmp < 0 || (cmp == 0 && split < x.split); 00255 } 00256 }; 00257 00258 MkTemplate::~MkTemplate() { } 00259 00260 void MkTemplate::setMatchExec(const string& me) { matchExec = me; } 00261 00262 void MkTemplate::debugRangeInfo(uint64 start, uint64 end, const char* msg, 00263 const PartialMatch* x) { 00264 printRangeInfo(start, end, msg, x); 00265 if (oldAreaEnd != start) debugRangeFailed(); 00266 oldAreaEnd = end; 00267 } 00268 00269 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
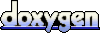