jigdo API
Zobstream Class Reference
Output stream which compresses the data sent to it before writing it to its final destination. More...
#include <zstream.hh>
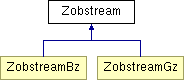
Public Member Functions | |
Zobstream (MD5Sum *md=0) | |
virtual | ~Zobstream () |
Calls close(), which might throw a Zerror exception! Call close() before destroying the object to avoid this. | |
bool | is_open () const |
void | close () |
Forces any remaining data to be compressed and written out. | |
bostream & | getStream () |
Get reference to underlying ostream. | |
Zobstream & | put (unsigned char x) |
Output 1 character. | |
Zobstream & | put (signed char x) |
Zobstream & | put (int x) |
Output the low 8 bits of an integer. | |
Zobstream & | put (char x) |
Zobstream & | put (uint32 x) |
Output 32 bit integer in little-endian order. | |
Zobstream & | write (const byte *x, unsigned n) |
Protected Member Functions | |
void | open (bostream &s, unsigned chunkLimit, unsigned todoBufSz) |
unsigned | chunkLim () const |
void | writeZipped (unsigned partId) |
virtual void | deflateEnd ()=0 |
virtual void | deflateReset ()=0 |
virtual unsigned | totalOut () const =0 |
virtual unsigned | totalIn () const =0 |
virtual unsigned | availOut () const =0 |
virtual unsigned | availIn () const =0 |
virtual byte * | nextOut () const =0 |
virtual byte * | nextIn () const =0 |
virtual void | setTotalOut (unsigned n)=0 |
virtual void | setTotalIn (unsigned n)=0 |
virtual void | setAvailOut (unsigned n)=0 |
virtual void | setAvailIn (unsigned n)=0 |
virtual void | setNextOut (byte *n)=0 |
virtual void | setNextIn (byte *n)=0 |
virtual void | zip2 (byte *start, unsigned len, bool finish)=0 |
Protected Attributes | |
ZipData * | zipBuf |
ZipData * | zipBufLast |
Static Protected Attributes | |
static const unsigned | ZIPDATA_SIZE = 64*1024 |
Classes | |
struct | ZipData |
Detailed Description
Output stream which compresses the data sent to it before writing it to its final destination.The b is for buffered - this class will buffer *all* generated output in memory until the bytes written to the underlying stream (i.e. the compressed data) exceed chunkLimit - once this happens, the zlib data is flushed and the resultant compressed chunk written out with a header (cf ../doc/TechDetails.txt for format). This is jigdo-specific.
Additional features mainly useful for jigdo: If an MD5Sum object is passed to Zobstream(), any data written to the output stream is also passed to the object.
Constructor & Destructor Documentation
Zobstream::Zobstream | ( | MD5Sum * | md = 0 |
) | [inline, explicit] |
virtual Zobstream::~Zobstream | ( | ) | [inline, virtual] |
Member Function Documentation
bool Zobstream::is_open | ( | ) | const [inline] |
Referenced by open(), write(), ZobstreamGz::zip2(), and ZobstreamBz::zip2().
void Zobstream::close | ( | ) |
bostream& Zobstream::getStream | ( | ) | [inline] |
Get reference to underlying ostream.
Zobstream & Zobstream::put | ( | unsigned char | x | ) | [inline] |
Output 1 character.
Zobstream & Zobstream::put | ( | signed char | x | ) | [inline] |
Zobstream & Zobstream::put | ( | int | x | ) | [inline] |
Output the low 8 bits of an integer.
Zobstream & Zobstream::put | ( | char | x | ) | [inline] |
Zobstream & Zobstream::put | ( | uint32 | x | ) |
Output 32 bit integer in little-endian order.
Zobstream & Zobstream::write | ( | const byte * | x, | |
unsigned | n | |||
) | [inline] |
void Zobstream::open | ( | bostream & | s, | |
unsigned | chunkLimit, | |||
unsigned | todoBufSz | |||
) | [inline, protected] |
References Assert, is_open(), and Paranoid.
Referenced by ZobstreamGz::open(), and ZobstreamBz::open().
unsigned Zobstream::chunkLim | ( | ) | const [inline, protected] |
Referenced by ZobstreamBz::open(), ZobstreamGz::zip2(), and ZobstreamBz::zip2().
void Zobstream::writeZipped | ( | unsigned | partId | ) | [protected] |
References _, buf, Zobstream::ZipData::data, debug, deflateReset(), Zobstream::ZipData::next, Paranoid, serialize4(), serialize6(), setAvailOut(), setNextOut(), setTotalIn(), setTotalOut(), totalIn(), totalOut(), MD5Sum::update(), writeBytes(), zipBuf, zipBufLast, and ZIPDATA_SIZE.
Referenced by ZobstreamGz::zip2(), and ZobstreamBz::zip2().
virtual void Zobstream::deflateEnd | ( | ) | [protected, pure virtual] |
Implemented in ZobstreamBz, and ZobstreamGz.
virtual void Zobstream::deflateReset | ( | ) | [protected, pure virtual] |
virtual unsigned Zobstream::totalOut | ( | ) | const [protected, pure virtual] |
virtual unsigned Zobstream::totalIn | ( | ) | const [protected, pure virtual] |
virtual unsigned Zobstream::availOut | ( | ) | const [protected, pure virtual] |
Implemented in ZobstreamBz, and ZobstreamGz.
virtual unsigned Zobstream::availIn | ( | ) | const [protected, pure virtual] |
Implemented in ZobstreamBz, and ZobstreamGz.
virtual byte* Zobstream::nextOut | ( | ) | const [protected, pure virtual] |
Implemented in ZobstreamBz, and ZobstreamGz.
virtual byte* Zobstream::nextIn | ( | ) | const [protected, pure virtual] |
Implemented in ZobstreamBz, and ZobstreamGz.
virtual void Zobstream::setTotalOut | ( | unsigned | n | ) | [protected, pure virtual] |
virtual void Zobstream::setTotalIn | ( | unsigned | n | ) | [protected, pure virtual] |
virtual void Zobstream::setAvailOut | ( | unsigned | n | ) | [protected, pure virtual] |
virtual void Zobstream::setAvailIn | ( | unsigned | n | ) | [protected, pure virtual] |
Implemented in ZobstreamBz, and ZobstreamGz.
virtual void Zobstream::setNextOut | ( | byte * | n | ) | [protected, pure virtual] |
virtual void Zobstream::setNextIn | ( | byte * | n | ) | [protected, pure virtual] |
Implemented in ZobstreamBz, and ZobstreamGz.
virtual void Zobstream::zip2 | ( | byte * | start, | |
unsigned | len, | |||
bool | finish | |||
) | [protected, pure virtual] |
Implemented in ZobstreamBz, and ZobstreamGz.
Member Data Documentation
const unsigned Zobstream::ZIPDATA_SIZE = 64*1024 [static, protected] |
Referenced by ZobstreamBz::deflateReset(), ZobstreamGz::open(), ZobstreamBz::open(), writeZipped(), ZobstreamGz::zip2(), and ZobstreamBz::zip2().
ZipData* Zobstream::zipBuf [protected] |
ZipData* Zobstream::zipBufLast [protected] |
Referenced by writeZipped(), ZobstreamGz::zip2(), and ZobstreamBz::zip2().
The documentation for this class was generated from the following files:
Generated on Tue Sep 23 14:27:43 2008 for jigdo by
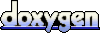