jigdo API
MD5Sum Class Reference
A 128-bit, cryptographically strong message digest algorithm. More...
#include <md5sum.hh>
Public Member Functions | |
MD5Sum () | |
Initialise the checksum. | |
MD5Sum (const MD5Sum &md) | |
Initialise with another checksum instance. | |
~MD5Sum () | |
MD5Sum & | operator= (const MD5Sum &md) |
Assign another checksum instance. | |
bool | operator== (const MD5Sum &md) const |
Tests for equality. | |
bool | operator!= (const MD5Sum &md) const |
bool | operator== (const MD5 &md) const |
bool | operator!= (const MD5 &md) const |
MD5Sum & | reset () |
Reset checksum object to the same state as immediately after its creation. | |
MD5Sum & | update (const byte *mem, size_t len) |
Process bytes with the checksum algorithm. | |
MD5Sum & | update (byte x) |
Add a single byte. NB, not implemented efficiently ATM. | |
MD5Sum & | finish () |
Process remaining bytes in internal buffer and create the final checksum. | |
MD5Sum & | finishForReuse () |
Exactly the same behaviour as finish(), but is more efficient if you are going to call reset() again in the near future to re-use the MD5Sum object. | |
MD5Sum & | abort () |
Deallocate buffers like finish(), but don't generate the final sum. | |
const byte * | digest () const |
Return 16 byte buffer with checksum. | |
INLINE string | toString () const |
Convert to string. | |
uint64 | updateFromStream (bistream &s, uint64 size, size_t bufSize=128 *1024, ProgressReporter &pr=noReport) |
Read data from file and update() this checksum with it. | |
template<class Iterator> | |
Iterator | serialize (Iterator i) const |
template<class ConstIterator> | |
ConstIterator | unserialize (ConstIterator i) |
size_t | serialSizeOf () const |
Friends | |
class | MD5 |
Classes | |
struct | md5_ctx |
class | ProgressReporter |
Class allowing JigdoCache to convey information back to the creator of a JigdoCache object. More... |
Detailed Description
A 128-bit, cryptographically strong message digest algorithm.Unless described otherwise, if a method returns an MD5Sum&, then this is a reference to the object itself, to allow chaining of calls.
Constructor & Destructor Documentation
MD5Sum::MD5Sum | ( | ) | [inline] |
Initialise the checksum.
MD5Sum::MD5Sum | ( | const MD5Sum & | md | ) |
MD5Sum::~MD5Sum | ( | ) | [inline] |
Member Function Documentation
bool MD5Sum::operator== | ( | const MD5Sum & | md | ) | const [inline] |
bool MD5Sum::operator== | ( | const MD5 & | md | ) | const [inline] |
bool MD5Sum::operator!= | ( | const MD5 & | md | ) | const [inline] |
MD5Sum & MD5Sum::reset | ( | ) | [inline] |
MD5Sum & MD5Sum::update | ( | const byte * | mem, | |
size_t | len | |||
) | [inline] |
Process bytes with the checksum algorithm.
May lead to some bytes being temporarily buffered internally.
References Paranoid.
Referenced by fileToImage(), main(), printBlockSums(), JigdoDescVec::put(), MkTemplate::run(), update(), update(), updateFromStream(), and Zobstream::writeZipped().
MD5Sum& MD5Sum::update | ( | byte | x | ) | [inline] |
MD5Sum & MD5Sum::finish | ( | ) | [inline] |
Process remaining bytes in internal buffer and create the final checksum.
- Returns:
- Pointer to the 16-byte checksum.
References Paranoid.
Referenced by fileToImage(), main(), and MkTemplate::run().
MD5Sum & MD5Sum::finishForReuse | ( | ) | [inline] |
Exactly the same behaviour as finish(), but is more efficient if you are going to call reset() again in the near future to re-use the MD5Sum object.
- Returns:
- Pointer to the 16-byte checksum.
References Paranoid.
Referenced by printBlockSums().
MD5Sum & MD5Sum::abort | ( | ) | [inline] |
Deallocate buffers like finish(), but don't generate the final sum.
const byte * MD5Sum::digest | ( | ) | const [inline] |
INLINE string MD5Sum::toString | ( | ) | const |
Convert to string.
uint64 MD5Sum::updateFromStream | ( | bistream & | s, | |
uint64 | size, | |||
size_t | bufSize = 128*1024 , |
|||
ProgressReporter & | pr = noReport | |||
) |
Read data from file and update() this checksum with it.
- Parameters:
-
s The stream to read from size Total number of bytes to read bufSize Size of temporary read buffer pr Reporter object
- Returns:
- Number of bytes read (==size if no error)
References buf, readBytes(), MD5Sum::ProgressReporter::readingMD5(), and update().
Referenced by main().
Iterator MD5Sum::serialize | ( | Iterator | i | ) | const [inline] |
References Paranoid, and MD5::serialize().
ConstIterator MD5Sum::unserialize | ( | ConstIterator | i | ) | [inline] |
References MD5::unserialize().
size_t MD5Sum::serialSizeOf | ( | ) | const [inline] |
References MD5::serialSizeOf().
Friends And Related Function Documentation
friend class MD5 [friend] |
The documentation for this class was generated from the following files:
- util/md5sum.hh
- util/glibc-md5.cc
- util/md5sum.cc
Generated on Tue Sep 23 14:27:43 2008 for jigdo by
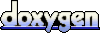