jigdo API
Last update by Admin on 2010-05-23
job/makeimagedl.hh
Go to the documentation of this file.00001 /* $Id: makeimagedl.hh,v 1.13 2003/09/16 23:32:10 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2003 | richard@ 00004 | \/�| Richard Atterer | atterer.net 00005 � '` � 00006 This program is free software; you can redistribute it and/or modify it 00007 under the terms of the GNU General Public License, version 2. See the file 00008 COPYING for details. 00009 00010 */ 00018 #ifndef MAKEIMAGEDL_HH 00019 #define MAKEIMAGEDL_HH 00020 00021 #include <config.h> 00022 00023 #include <glib.h> 00024 #include <sys/stat.h> 00025 #include <sys/types.h> 00026 00027 #include <string> 00028 00029 #include <datasource.hh> 00030 #include <ilist.hh> 00031 #include <jigdo-io.fh> 00032 #include <job.hh> 00033 #include <makeimage.hh> 00034 #include <makeimagedl.fh> 00035 #include <md5sum.hh> 00036 #include <nocopy.hh> 00037 #include <single-url.hh> 00038 #include <status.hh> 00039 #include <url-mapping.hh> 00040 //______________________________________________________________________ 00041 00067 class Job::MakeImageDl : NoCopy /*, public JigdoConfig::ProgressReporter*/ { 00068 public: 00069 class Child; 00070 friend class Child; 00071 class ChildListBase; 00072 class IO; 00073 IOSource<IO> io; // Points to e.g. a GtkMakeImage 00074 //____________________ 00075 00078 static const int MAX_INCLUDES = 100; 00079 00080 enum State { 00081 DOWNLOADING_JIGDO, 00082 DOWNLOADING_TEMPLATE, 00083 FINAL_STATE, // Value isn't actually used; all below are final states: 00084 ERROR 00085 }; 00086 00089 //inline static const char* destDescTemplate(); 00090 00095 MakeImageDl(/*IO* ioPtr,*/const string& jigdoUri, const string& destination); 00096 00098 ~MakeImageDl(); 00099 00101 void killAllChildren(); 00102 00105 void run(); 00106 00107 inline const string& jigdoUri() const; 00108 00110 inline State state() const; 00111 00114 inline const string& tmpDir() const; 00115 00117 inline void generateError(const string& message, State newState = ERROR); 00119 inline bool finalState() const; 00120 00121 #if 0 00122 00131 void childSucceeded(Child* childDl, DataSource::IO* childIo/*, 00132 DataSource::IO* frontend*/); 00133 #endif 00134 00139 void childFailed(Child* childDl); 00140 00143 void setImageSection(string* imageName, string* imageInfo, 00144 string* imageShortInfo, PartUrlMapping* templateUrls, 00145 MD5** templateMd5); 00148 inline bool haveImageSection() const; 00149 00151 UrlMap urlMap; 00152 00174 Child* childFor(const string& url, const MD5* md = 0, 00175 string* leafnameOut = 0, Child* reuseChild = 0); 00176 00180 inline Child* childFor(PartUrlMapping* urls, const MD5* md = 0); 00181 00182 typedef IList<ChildListBase> ChildList; 00184 inline const ChildList& children() const; 00185 00188 inline const string& imageName() const { return imageNameVal; } 00189 inline const string& imageShortInfo() const { return imageShortInfoVal; } 00190 //inline const string& templateUrl() const { return templateUrlVal; } 00191 inline const MD5* templateMd5() const { return templateMd5Val; } 00221 void imageInfo(string* output, bool escapedText, 00222 const char* subst[13]) const; 00224 enum { B, B_, I, I_, TT, TT_, U, U_, BIG, BIG_, SMALL, SMALL_, BR }; 00225 00228 inline const string& imageInfoOrig() const { return imageInfoVal; } 00229 00233 void jigdoFinished(); 00234 00235 private: // To be called by Child only: 00236 00237 /* Called by Child when the download has succeeded: Rename a '~' download 00238 to '-' to indicate that it is complete. */ 00239 void singleUrlFinished(Child* c); 00240 /* Called by Child when the download has succeeded, but the expected 00241 content checksum was wrong: Rename from c~ContentChecksum to 00242 u-UrlChecksum */ 00243 void singleUrlWrongContent(Child* c); 00244 00245 // Called from Child::job_succeeded() when the template d/l has finished 00246 void templateFinished(); 00247 00248 private: // Really private 00249 00250 // Write a ReadMe.txt to the download dir; fails silently 00251 void writeReadMe(); 00252 00253 // Starts the initial .jigdo download 00254 void createJigdoDownload(); 00255 00256 /* Return filename for content md5sum cache entry: 00257 "/home/x/jigdo-blasejfwe/c-nGJ2hQpUNCIZ0fafwQxZmQ" 00258 @param leafnameOut String to assign cache entry leafname, or null 00259 @param isFinished true to use "-", false to use "~" 00260 @param isC true to use "c", false to use "u" */ 00261 string cachePathnameContent(const MD5& md, string* leafnameOut = 0, 00262 bool isFinished = true, bool isC = true); 00263 /* Return filename for URL cache entry: 00264 "/home/x/jigdo-blasejfwe/u-nGJ2hQpUNCIZ0fafwQxZmQ" 00265 @param leafnameOut String to assign cache entry leafname, or null 00266 @param isFinished true to use "-", false to use "~" */ 00267 string cachePathnameUrl(const string& url, string* leafnameOut = 0, 00268 bool isFinished = true); 00269 // Add leafname for object to arg string, e.g. "u-nGJ2hQpUNCIZ0fafwQxZmQ" 00270 //static void appendLeafname(string* s, bool contentMd, const MD5& md); 00271 /* Turn the '-' in string created by above function into a '~' or v.v. 00272 Works even if leafname is preceded by dirname or similar in s */ 00273 static inline void toggleLeafname(string* s); 00274 00275 // Helper methods for childFor() 00276 Child* childForCompletedUrl(const struct stat& fileInfo, 00277 const string& filename, const MD5* md, Child* reuseChild); 00278 Child* childForCompletedContent(const struct stat& fileInfo, 00279 const string& filename, const MD5* md, Child* reuseChild); 00280 Child* childForSemiCompleted(const struct stat& fileInfo, 00281 const string& filename, Child* reuseChild); 00282 00283 //static const char* destDescTemplateVal; 00284 00285 State stateVal; // State, e.g. "downloading jigdo file", "error" 00286 00287 string jigdoUrl; // URL of .jigdo file 00288 Job::JigdoIO* jigdoIo; // toplevel JigdoIO, while state == DOWNLOADING_JIGDO 00289 ChildList childrenVal; // Child downloads 00290 00291 string dest; // Destination dir. No trailing '/', empty string for root dir 00292 string tmpDirVal; // Temporary dir, a subdir of dest 00293 00294 // Workhorse which actually generates the image from the data we feed it 00295 MakeImage mi; 00296 00297 // Info about first image section of this .jigdo, if any 00298 string imageNameVal; 00299 string imageInfoVal, imageShortInfoVal; 00300 SmartPtr<PartUrlMapping> templateUrls; // Can contain a list of altern. URLs 00301 MD5* templateMd5Val; 00302 00303 static gboolean jigdoFinished_callback(gpointer); 00304 void jigdoFinished2(); 00305 int callbackId; // glib callback function ID 00306 }; 00307 //______________________________________________________________________ 00308 00310 class Job::MakeImageDl::IO : public Job::IO { 00311 public: 00312 00316 virtual void job_deleted() = 0; 00317 00319 virtual void job_succeeded() = 0; 00320 00323 virtual void job_failed(const string& message) = 0; 00324 00326 virtual void job_message(const string& message) = 0; 00327 00328 #if 0 00329 00342 // virtual Job::DataSource::IO* makeImageDl_new( 00343 // Job::DataSource* childDownload, const string& uri, 00344 // const string& destDesc) = 0; 00345 #endif 00346 00359 virtual void makeImageDl_new(Job::DataSource* childDownload, 00360 const string& uri, const string& destDesc) = 0; 00361 00364 virtual void makeImageDl_finished(Job::DataSource* childDownload) = 0; 00365 00369 virtual void makeImageDl_haveImageSection() = 0; 00370 }; 00371 //______________________________________________________________________ 00372 00376 class Job::MakeImageDl::ChildListBase : public IListBase { 00377 friend class Job::MakeImageDl::Child; 00378 explicit ChildListBase() { } // Private ctor, only usable by Child 00379 public: 00383 inline Child* get(); 00384 inline const Child* get() const; 00385 }; 00386 //____________________ 00387 00400 class Job::MakeImageDl::Child 00401 : NoCopy, public ChildListBase, public Job::DataSource::IO { 00402 friend class MakeImageDl; 00403 public: 00404 inline ~Child(); 00405 00407 MakeImageDl* master() const { return masterVal; } 00409 inline DataSource* source() const; 00411 inline void deleteSource(); 00413 //inline DataSource::IO* childIo() const; 00414 00421 inline Child(MakeImageDl* m, ChildList* listHead, 00422 DataSource* src, const MD5* expectedContent); 00424 //inline void setChildIo(DataSource::IO* c); 00425 00426 # if DEBUG 00427 // childFailed() or childSucceeded() MUST always be called for a Child 00428 bool childSuccFail; 00429 # endif 00430 00431 private: 00432 00433 // (Re)initialize data members, except for urls and lastUrl. Returns this 00434 inline Child* init(MakeImageDl* m, ChildList* list, 00435 DataSource* src, const MD5* expectedContent); 00436 00437 // Virtual methods from DataSource::IO. 00438 virtual void job_deleted(); 00439 virtual void job_succeeded(); 00440 virtual void job_failed(const string& message); 00441 virtual void job_message(const string& message); 00442 virtual void dataSource_dataSize(uint64 n); 00443 virtual void dataSource_data(const byte* data, unsigned size, 00444 uint64 currentSize); 00445 MakeImageDl* masterVal; 00446 DataSource* sourceVal; 00447 bool checkContent; // True iff checksum of downloaded data is to be verified 00448 MD5 md; // Only if contentMd==true, EXPECTED checksum of the download data 00449 MD5Sum mdCheck; // Only if contentMd==true, used to calculate actual checksum 00450 SmartPtr<PartUrlMapping> urls; // Null if only a single URL (.jigdo d/l) 00451 vector<UrlMapping*>* lastUrl; // To record last URL output by templateUrls 00452 }; 00453 //====================================================================== 00454 00455 // const char* Job::MakeImageDl::destDescTemplate() { 00456 // return destDescTemplateVal; 00457 // } 00458 00459 const string& Job::MakeImageDl::jigdoUri() const { return jigdoUrl; } 00460 00461 Job::MakeImageDl::State Job::MakeImageDl::state() const { return stateVal; } 00462 00463 void Job::MakeImageDl::generateError(const string& message, State newState) { 00464 if (finalState()) return; 00465 stateVal = newState; 00466 IOSOURCE_SEND(IO, io, job_failed, (message)); 00467 } 00468 00469 bool Job::MakeImageDl::finalState() const { return state() > FINAL_STATE; } 00470 00471 const string& Job::MakeImageDl::tmpDir() const { return tmpDirVal; } 00472 00473 void Job::MakeImageDl::toggleLeafname(string* s) { 00474 int off = s->length() - 23; 00475 Paranoid((*s)[off] == '-' || (*s)[off] == '~'); 00476 (*s)[off] ^= ('-' ^ '~'); 00477 } 00478 00479 bool Job::MakeImageDl::haveImageSection() const { 00480 return !imageNameVal.empty(); 00481 } 00482 00483 const Job::MakeImageDl::ChildList& Job::MakeImageDl::children() const { 00484 return childrenVal; 00485 } 00486 00487 Job::MakeImageDl::Child* Job::MakeImageDl::childFor(PartUrlMapping* urls, 00488 const MD5* md) { 00489 auto_ptr<vector<UrlMapping*> > lastUrl(new vector<UrlMapping*>); 00490 string url = urls->enumerate(lastUrl.get()); 00491 Child* c = childFor(url, md); 00492 if (c != 0) { 00493 c->urls = urls; 00494 c->lastUrl = lastUrl.release(); 00495 } 00496 return c; 00497 } 00498 //____________________ 00499 00501 Job::MakeImageDl::Child* Job::MakeImageDl::ChildListBase::get() 00502 { return static_cast<MakeImageDl::Child*>(this); } 00503 const Job::MakeImageDl::Child* Job::MakeImageDl::ChildListBase::get() const 00504 { return static_cast<const MakeImageDl::Child*>(this); } 00505 00506 Job::MakeImageDl::Child* Job::MakeImageDl::Child::init(MakeImageDl* m, 00507 ChildList* /*list*/, DataSource* src, const MD5* expectedContent) { 00508 masterVal = m; 00509 sourceVal = src; 00510 checkContent = (expectedContent != 0); 00511 if (expectedContent != 0) md = *expectedContent; else md.clear(); 00512 # if DEBUG 00513 childSuccFail = false; 00514 # endif 00515 // Add ourself as listener to DataSource 00516 src->io.addListener(*implicit_cast<DataSource::IO*>(this)); 00517 return this; 00518 } 00519 00520 Job::MakeImageDl::Child::Child(MakeImageDl* m, ChildList* list, 00521 DataSource* src, const MD5* expectedContent) 00522 : ChildListBase(), Job::DataSource::IO(), 00523 md(), mdCheck(), urls(), lastUrl(0) { 00524 Paranoid(list != 0); 00525 init(m, list, src, expectedContent); 00526 // Add ourself to parent's list of children 00527 list->push_back(*implicit_cast<ChildListBase*>(this)); 00528 //msg("Child %1", this); 00529 } 00530 00531 Job::MakeImageDl::Child::~Child() { 00532 # if DEBUG 00533 //msg("~Child %1", this); 00534 Paranoid(childSuccFail); 00535 # endif 00536 deleteSource(); 00537 delete lastUrl; 00538 } 00539 00540 Job::DataSource* Job::MakeImageDl::Child::source() const { 00541 return sourceVal; 00542 } 00543 00544 void Job::MakeImageDl::Child::deleteSource() { 00545 delete sourceVal; 00546 sourceVal = 0; 00547 } 00548 00549 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
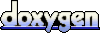