jigdo API
MessageBox Class Reference
Display an error box with a message and standard or user-supplied buttons. More...
#include <messagebox.hh>

Public Types | |
enum | { NONE = 0, HELP = 1 << 0, OK = 1 << 1, CLOSE = 1 << 2, CANCEL = 1 << 3 } |
typedef void(* | ResponseHandler )(GtkDialog *, int, gpointer) |
implResponseHandler(GtkDialog* diag, int response, gpointer data) | |
Public Member Functions | |
MessageBox (const char *type, int buttons, const char *heading, const char *message=0) | |
Displays message in a GtkMessageDialog - when the user clicks the OK button, the window is closed again. | |
MessageBox (const char *type, int buttons, const string &heading, const char *message) | |
As above, but with string& instead of char*. | |
MessageBox (const char *type, int buttons, const char *heading, const string &message) | |
As above, but with string& instead of char*. | |
MessageBox (const char *type, int buttons, const string &heading) | |
As above, but with string& instead of char*. | |
MessageBox (const char *type, int buttons, const string &heading, const string &message) | |
As above, but with string& instead of char*. | |
~MessageBox () | |
MessageBox * | show () |
Open the dialog box. | |
MessageBox * | show_noAutoClose () |
As above, but don't close when a button is pressed. | |
GtkWidget * | addButton (const char *buttonText, int response) |
Add a text button to the dialog. | |
GtkWidget * | addButton (const char *buttonText, GtkResponseType response) |
GtkWidget * | addStockButton (const char *buttonType, int response) |
Add a standard button to the dialog. | |
GtkWidget * | addStockButton (const char *buttonType, GtkResponseType response) |
MessageBox * | onResponse (ResponseHandler handler, gpointer data) |
You must take care yourself to be delivered signals when the user clicks on a button. | |
Static Public Attributes | |
static const char *const | MESSAGE = "gtk-dialog-info" |
static const char *const | INFO = "gtk-dialog-info" |
static const char *const | WARNING = "gtk-dialog-warning" |
static const char *const | QUESTION = "gtk-dialog-question" |
static const char *const | ERROR = "gtk-dialog-error" |
Friends | |
class | Ref |
Classes | |
class | Ref |
Ref object to store reference to a MessageBox in. More... |
Detailed Description
Display an error box with a message and standard or user-supplied buttons.
Member Typedef Documentation
typedef void(* MessageBox::ResponseHandler)(GtkDialog *, int, gpointer) |
implResponseHandler(GtkDialog* diag, int response, gpointer data)
- Parameters:
-
diag response Code of response. Either one of the responses you registered, or GTK_RESPONSE_DELETE_EVENT if the user clicked on the window's close icon. If the user presses the Escape key, either any GTK_RESPONSE_CANCEL (if present) is simulated as pressed, or the only button of the dialog is simulated as pressed (if there is only one), or nothing happens (i.e. >1 buttons and none of them is GTK_RESPONSE_CANCEL). data The pointer passed to onResponse().
Member Enumeration Documentation
Constructor & Destructor Documentation
MessageBox::MessageBox | ( | const char * | type, | |
int | buttons, | |||
const char * | heading, | |||
const char * | message = 0 | |||
) | [inline] |
Displays message in a GtkMessageDialog - when the user clicks the OK button, the window is closed again.
Any number of independent error boxes can be open at the same time.
For non-standard buttons, use GTK_BUTTONS_NONE, then addButton().
heading and message must be valid UTF-8. Markup is not escaped, do this yourself if necessary. message is optional.
- Parameters:
-
type Type of icon to display buttons Or'ed together: HELP, OK, CLOSE, CANCEL. HELP is special in that the button is added at the left side of the dialog. OK is special because a default signal handler is added which closes the dialog. heading Error text to be printed in big font at top of dialog, or null if only message is to be displayed. message Main error message
MessageBox::MessageBox | ( | const char * | type, | |
int | buttons, | |||
const string & | heading, | |||
const char * | message | |||
) | [inline] |
As above, but with string& instead of char*.
MessageBox::MessageBox | ( | const char * | type, | |
int | buttons, | |||
const char * | heading, | |||
const string & | message | |||
) | [inline] |
As above, but with string& instead of char*.
MessageBox::MessageBox | ( | const char * | type, | |
int | buttons, | |||
const string & | heading | |||
) | [inline] |
As above, but with string& instead of char*.
MessageBox::MessageBox | ( | const char * | type, | |
int | buttons, | |||
const string & | heading, | |||
const string & | message | |||
) | [inline] |
As above, but with string& instead of char*.
MessageBox::~MessageBox | ( | ) |
References Assert, debug, MessageBox::Ref::get(), and MessageBox::Ref::messageBox.
Member Function Documentation
MessageBox * MessageBox::show | ( | ) | [inline] |
Open the dialog box.
(This isn't done in the ctor because if you add your own buttons after opening it, its size changes, which may cause the window manager to display it partially off-screen.) Also registers a callback which closes the window once a button is pressed, *except* when that button causes a response GTK_RESPONSE_HELP
- Returns:
- "this"
References show_noAutoClose().
Referenced by JobLine::create(), GtkSingleUrl::on_closeButton_clicked(), GtkSingleUrl::on_restartButton_clicked(), GtkSingleUrl::on_startButton_clicked(), and GtkSingleUrl::run().
MessageBox * MessageBox::show_noAutoClose | ( | ) |
GtkWidget * MessageBox::addButton | ( | const char * | buttonText, | |
int | response | |||
) |
Add a text button to the dialog.
- Returns:
- The new button
Referenced by addButton(), JobLine::create(), GtkSingleUrl::on_closeButton_clicked(), GtkSingleUrl::on_restartButton_clicked(), and GtkSingleUrl::on_startButton_clicked().
GtkWidget * MessageBox::addButton | ( | const char * | buttonText, | |
GtkResponseType | response | |||
) | [inline] |
References addButton().
GtkWidget * MessageBox::addStockButton | ( | const char * | buttonType, | |
int | response | |||
) |
Add a standard button to the dialog.
- Parameters:
-
buttonType e.g. "gtk-cancel" response e.g. GTK_RESPONSE_CANCEL, or a positive value of your choice
- Returns:
- The new button
Referenced by addStockButton(), GtkSingleUrl::on_closeButton_clicked(), GtkSingleUrl::on_restartButton_clicked(), and GtkSingleUrl::on_startButton_clicked().
GtkWidget * MessageBox::addStockButton | ( | const char * | buttonType, | |
GtkResponseType | response | |||
) | [inline] |
References addStockButton().
MessageBox * MessageBox::onResponse | ( | ResponseHandler | handler, | |
gpointer | data | |||
) | [inline] |
You must take care yourself to be delivered signals when the user clicks on a button.
The MessageBox object is deleted when the dialog is destroyed - usually, this happens automatically, but it won't happen for a click on a "Help" button and if you used show_noAutoClose() instead of show().
- Returns:
- "this"
Referenced by GtkSingleUrl::on_closeButton_clicked(), GtkSingleUrl::on_restartButton_clicked(), and GtkSingleUrl::on_startButton_clicked().
Friends And Related Function Documentation
friend class Ref [friend] |
Member Data Documentation
const char *const MessageBox::MESSAGE = "gtk-dialog-info" [static] |
const char *const MessageBox::INFO = "gtk-dialog-info" [static] |
Referenced by JobLine::create().
const char *const MessageBox::WARNING = "gtk-dialog-warning" [static] |
const char *const MessageBox::QUESTION = "gtk-dialog-question" [static] |
const char *const MessageBox::ERROR = "gtk-dialog-error" [static] |
Referenced by JobLine::create(), GtkSingleUrl::on_startButton_clicked(), and GtkSingleUrl::run().
The documentation for this class was generated from the following files:
- gtk/messagebox.hh
- gtk/messagebox.cc
Generated on Tue Sep 23 14:27:43 2008 for jigdo by
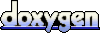