jigdo API
Last update by Admin on 2010-05-23
cachefile.hh
Go to the documentation of this file.00001 /* $Id: cachefile.hh,v 1.10 2003/03/03 20:47:16 richard Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2001-2002 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00011 00045 #ifndef CACHEFILE_HH 00046 #define CACHEFILE_HH 00047 00048 #include <config.h> 00049 00050 #include <string> 00051 #include <time.h> /* for time_t */ 00052 00053 #if HAVE_LIBDB 00054 #include <db.h> 00055 #include <stdlib.h> /* free() */ 00056 #include <string.h> /* memcpy(), memset() */ 00057 00058 #include <debug.hh> 00059 #include <status.hh> 00060 //______________________________________________________________________ 00061 00063 struct DbError : public Error { 00064 explicit DbError(int c) : Error(db_strerror(c)), code(c) { } 00065 DbError(int c, const string& m) : Error(m), code(c) { } 00066 DbError(int c, const char* m) : Error(m), code(c) { } 00067 int code; 00068 }; 00069 //______________________________________________________________________ 00070 00072 class CacheFile { 00073 public: 00075 CacheFile(const char* dbName); 00076 inline ~CacheFile(); 00077 00085 Status find(const byte*& resultData, size_t& resultSize, 00086 const string& fileName, uint64 fileSize, time_t mtime); 00087 00095 Status findName(const byte*& resultData, size_t& resultSize, 00096 const string& fileName, 00097 off_t& resultFileSize, time_t& resultMtime); 00098 00102 inline void insert(const byte* inData, size_t inSize, 00103 const string& fileName, time_t mtime, uint64 fileSize); 00107 template<class Functor> 00108 inline void insert(Functor f, size_t inSize, const string& fileName, 00109 time_t mtime, uint64 fileSize); 00110 00113 void expire(time_t t); 00114 00115 private: 00116 // Don't copy 00117 explicit inline CacheFile(const CacheFile&); 00118 inline CacheFile& operator=(const CacheFile&); 00119 byte* insert_prepare(size_t inSize); 00120 void insert_perform(const string& fileName, time_t mtime, uint64 fileSize); 00121 00122 /* Byte offsets of first members of a cache entry (see start of this 00123 file). Defining a struct with byte members would be more 00124 convenient, but would also be a recipe for disaster because of 00125 alignment problems. (E.g., a RISC machine might pad to the next 00126 multiple of 4 bytes after every byte member.) */ 00127 enum { ACCESS = 0, MTIME = 4, SIZE = 8, USER_DATA = 14 }; 00128 00129 DB* db; // Database object 00130 DBT data; // Object for result data 00131 }; 00132 //______________________________________________________________________ 00133 00134 CacheFile::~CacheFile() { 00135 free(data.data); 00136 Paranoid(db != 0); 00137 db->close(db, 0); // no flags, ignore any errors 00138 } 00139 //______________________________________________________________________ 00140 00141 void CacheFile::insert(const byte* inData, size_t inSize, 00142 const string& fileName, time_t mtime, uint64 fileSize) { 00143 memcpy(insert_prepare(inSize), inData, inSize); 00144 insert_perform(fileName, mtime, fileSize); 00145 } 00146 00147 template<class Functor> 00148 void CacheFile::insert(Functor f, size_t inSize, const string& fileName, 00149 time_t mtime, uint64 fileSize) { 00150 f(insert_prepare(inSize)); 00151 insert_perform(fileName, mtime, fileSize); 00152 } 00153 00154 //====================================================================== 00155 00156 #else 00157 00158 // !HAVE_LIBDB - provide a dummy implementation which does nothing 00159 00160 class CacheFile { 00161 public: 00162 CacheFile(const char*) { } 00163 ~CacheFile() { } 00164 bool find(const byte*&, size_t&, const string&, time_t, uint64) { 00165 return false; 00166 } 00167 bool find_name(const byte*&, size_t&, const string&, 00168 long long int&, time_t&) { 00169 return false; 00170 } 00171 template<class Functor> 00172 void insert(Functor, size_t, const string&, time_t, uint64) { } 00173 void insert(const byte*, size_t, const string&, time_t, uint64) { } 00174 void expire(time_t) { } 00175 }; 00176 00177 #endif 00178 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
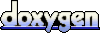