jigdo API
Last update by Admin on 2010-05-23
partialmatch.hh
Go to the documentation of this file.00001 /* $Id: partialmatch.hh,v 1.1.1.1 2003/07/04 22:29:14 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2002 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00016 #ifndef PARTIALMATCH_HH 00017 #include <debug.hh> 00018 #include <log.hh> 00019 #define PARTIALMATCH_HH 00020 00021 #ifndef INLINE 00022 # ifdef NOINLINE 00023 # define INLINE 00024 # else 00025 # define INLINE inline 00026 # endif 00027 #endif 00028 00032 class MkTemplate::PartialMatch { 00033 friend class MkTemplate::PartialMatchQueue; 00034 public: 00036 uint64 startOffset() const { return startOff; } 00037 void setStartOffset(uint64 o) { startOff = o; } 00038 00040 uint64 nextEvent() const { return nextEv; } 00043 INLINE void setNextEvent(PartialMatchQueue* matches, uint64 newNextEvent); 00044 00046 size_t blockOffset() const { return blockOff; } 00047 void setBlockOffset(size_t b) { blockOff = b; } 00048 00050 size_t blockNumber() const { return blockNr; } 00051 void setBlockNumber(size_t b) { blockNr = b; } 00052 00054 FilePart* file() const { return filePart; } 00055 void setFile(FilePart* f) { filePart = f; } 00056 00058 PartialMatch* next() const { return nextPart; } 00059 00060 private: 00061 PartialMatch() { } // Only to be instantiated by PartialMatchQueue 00062 uint64 startOff; // Offset in image at which this match starts 00063 uint64 nextEv; // Next value of off at which to finish() sum & compare 00064 size_t blockOff; // Offset in buf of start of current MD5 block 00065 size_t blockNr; // Number of block in file, i.e. index into file->sums[] 00066 FilePart* filePart; // File whose sums matched so far 00067 PartialMatch* nextPart; 00068 bool operator<=(const PartialMatch& x) { 00069 return nextEvent() <= x.nextEvent(); 00070 } 00071 }; 00072 //________________________________________ 00073 00077 class MkTemplate::PartialMatchQueue { 00078 friend class MkTemplate::PartialMatch; 00079 public: 00080 inline PartialMatchQueue(); 00081 00082 bool empty() const { return head == 0; } 00083 00084 bool full() const { return freeHead == 0; } 00085 00086 /* If true is returned, the queue is not only full, findDropCandidate() is 00087 also going to always return 0 from now on. Intended to be used for 00088 optimisation: As long as crammed()==true, needn't even check for 00089 further prospective matches. 00090 The value returned by this function is set to true by 00091 findDropCandidate(), and set to false by erase(), eraseFront(), 00092 eraseStartOffsetLess() and setNextEvent(). (NB not by 00093 setStartOffset()) */ 00094 //bool crammed() const { return crammedVal; } 00095 00096 PartialMatch* front() const { return head; } 00097 00102 INLINE PartialMatch* addFront(); 00103 00106 INLINE PartialMatch* lowestStartOffset() const; 00107 00111 INLINE uint64 nextEvent() const; 00112 00115 INLINE PartialMatch* findStartOffset(uint64 off) const; 00116 00119 INLINE PartialMatch* findLowestStartOffset() const; 00120 00122 inline void erase(); 00123 00125 INLINE void eraseFront(); 00126 00128 INLINE void eraseStartOffsetLess(uint64 off); 00129 00137 INLINE PartialMatch* findDropCandidate(unsigned* sectorLength, 00138 uint64 newStartOffset); 00139 00140 # if DEBUG 00141 void consistencyCheck() const; 00142 # else 00143 void consistencyCheck() const { } 00144 # endif 00145 00146 private: 00147 /* The size of the linked list of MD5 blocks awaiting matching must 00148 be limited for cases where there are lots of overlapping matches, 00149 e.g. both image and a file are all zeroes. */ 00150 static const int MAX_MATCHES = 2048; 00151 PartialMatch* head; // First entry of queue, or null if queue empty 00152 PartialMatch* freeHead; // First elem of linked list of free slots, or null 00153 PartialMatch data[MAX_MATCHES]; 00154 00155 /* If true, queue is full, and furthermore findDropCandidate() will always 00156 return 0 */ 00157 //bool crammedVal; 00158 }; 00159 //______________________________________________________________________ 00160 00161 void MkTemplate::PartialMatchQueue::erase() { 00162 head = 0; 00163 freeHead = &data[0]; 00164 //crammedVal = false; 00165 // Put all elements in free list 00166 data[MAX_MATCHES - 1].nextPart = 0; 00167 for (int i = MAX_MATCHES - 2; i >= 0; --i) 00168 data[i].nextPart = &data[i + 1]; 00169 consistencyCheck(); 00170 } 00171 00172 MkTemplate::PartialMatchQueue::PartialMatchQueue() { 00173 erase(); 00174 } 00175 //______________________________________________________________________ 00176 00177 #ifndef NOINLINE 00178 # include <partialmatch.ih> /* NOINLINE */ 00179 #endif 00180 00181 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
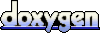