jigdo API
Last update by Admin on 2010-05-23
util/autonullptr.hh File Reference
A pointer which gets set to null if the pointed-to object is deleted. More...
#include <ilist.hh>
Go to the source code of this file.
Classes | |
class | AutoNullPtr< T > |
A pointer which gets set to null if the pointed-to object is deleted. More... | |
class | AutoNullPtrBase< T > |
Derive from this class to make your class instances referenceable by AutoNullPtr. More... | |
Functions | |
The regular pointer tests | |
template<class T> | |
bool | operator== (const AutoNullPtr< T > &a, const T *b) |
template<class T> | |
bool | operator== (const T *b, const AutoNullPtr< T > &a) |
template<class T> | |
bool | operator== (const AutoNullPtr< T > &a, const AutoNullPtr< T > &b) |
template<class T> | |
bool | operator!= (const AutoNullPtr< T > &a, const T *b) |
template<class T> | |
bool | operator!= (const T *b, const AutoNullPtr< T > &a) |
template<class T> | |
bool | operator!= (const AutoNullPtr< T > &a, const AutoNullPtr< T > &b) |
template<class T> | |
bool | operator< (const AutoNullPtr< T > &a, const T *b) |
template<class T> | |
bool | operator< (const T *b, const AutoNullPtr< T > &a) |
template<class T> | |
bool | operator< (const AutoNullPtr< T > &a, const AutoNullPtr< T > &b) |
template<class T> | |
bool | operator> (const AutoNullPtr< T > &a, const T *b) |
template<class T> | |
bool | operator> (const T *b, const AutoNullPtr< T > &a) |
template<class T> | |
bool | operator> (const AutoNullPtr< T > &a, const AutoNullPtr< T > &b) |
template<class T> | |
bool | operator<= (const AutoNullPtr< T > &a, const T *b) |
template<class T> | |
bool | operator<= (const T *b, const AutoNullPtr< T > &a) |
template<class T> | |
bool | operator<= (const AutoNullPtr< T > &a, const AutoNullPtr< T > &b) |
template<class T> | |
bool | operator>= (const AutoNullPtr< T > &a, const T *b) |
template<class T> | |
bool | operator>= (const T *b, const AutoNullPtr< T > &a) |
template<class T> | |
bool | operator>= (const AutoNullPtr< T > &a, const AutoNullPtr< T > &b) |
Detailed Description
A pointer which gets set to null if the pointed-to object is deleted.Somewhat equivalent to "weak references" in Java.
class MyClass : public AutoNullPtrBase<MyClass> { ... your class members here ... } myClass; AutoNullPtr<MyClass> ptr = &myClass;
Implementation: AutoNullPtrBase contains the head of a linked list of AutoNullPtrs, sets them all to 0 from its dtor. ~AutoNullPtr removes itself from that list.
Function Documentation
template<class T>
bool operator!= | ( | const AutoNullPtr< T > & | a, | |
const AutoNullPtr< T > & | b | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator!= | ( | const T * | b, | |
const AutoNullPtr< T > & | a | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator!= | ( | const AutoNullPtr< T > & | a, | |
const T * | b | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator< | ( | const AutoNullPtr< T > & | a, | |
const AutoNullPtr< T > & | b | |||
) | [inline] |
template<class T>
bool operator< | ( | const T * | b, | |
const AutoNullPtr< T > & | a | |||
) | [inline] |
template<class T>
bool operator< | ( | const AutoNullPtr< T > & | a, | |
const T * | b | |||
) | [inline] |
template<class T>
bool operator<= | ( | const AutoNullPtr< T > & | a, | |
const AutoNullPtr< T > & | b | |||
) | [inline] |
template<class T>
bool operator<= | ( | const T * | b, | |
const AutoNullPtr< T > & | a | |||
) | [inline] |
template<class T>
bool operator<= | ( | const AutoNullPtr< T > & | a, | |
const T * | b | |||
) | [inline] |
template<class T>
bool operator== | ( | const AutoNullPtr< T > & | a, | |
const AutoNullPtr< T > & | b | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator== | ( | const T * | b, | |
const AutoNullPtr< T > & | a | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator== | ( | const AutoNullPtr< T > & | a, | |
const T * | b | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator> | ( | const AutoNullPtr< T > & | a, | |
const AutoNullPtr< T > & | b | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator> | ( | const T * | b, | |
const AutoNullPtr< T > & | a | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator> | ( | const AutoNullPtr< T > & | a, | |
const T * | b | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator>= | ( | const AutoNullPtr< T > & | a, | |
const AutoNullPtr< T > & | b | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator>= | ( | const T * | b, | |
const AutoNullPtr< T > & | a | |||
) | [inline] |
References AutoNullPtr< T >::get().
template<class T>
bool operator>= | ( | const AutoNullPtr< T > & | a, | |
const T * | b | |||
) | [inline] |
References AutoNullPtr< T >::get().
Generated on Tue Sep 23 14:27:42 2008 for jigdo by
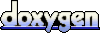