jigdo API
Download Class Reference
Class containing the state of a download. More...
#include <download.hh>
Public Member Functions | |
Download (const string &uri, Output *o) | |
~Download () | |
void | setResumeOffset (uint64 offset) |
Set offset to resume from - download must not yet have been started, call before run(). | |
uint64 | resumeOffset () const |
Value passed to setResumeOffset(). | |
void | run () |
Whether to send a "Pragma: no-cache" header. | |
const string & | uri () const |
void | pause () |
Pause the request. | |
void | cont () |
Continue with the download. | |
bool | paused () const |
Is the download paused? (i.e. | |
bool | failed () const |
Is the download paused, or is it not yet paused, but will be soon? | |
bool | succeeded () const |
Is download finished? (Also returns true if FTP/HTTP1.0 connection dropped). | |
bool | interrupted () const |
The connection was interrupted during the download, or it timed out. | |
void | stop () |
Forcibly stop this download. | |
Output * | output () const |
Return the Output object. | |
void | setOutput (Output *o) |
Set the Output object. | |
Static Public Member Functions | |
static void | init () |
Initialize libwww - call this before starting any downloads. | |
static void | cleanup () |
Clean up - call this after all requests are finished. | |
Classes | |
class | Output |
A derived class of Output must be supplied by the user of Download, to take care of the downloaded data and to output progress reports. More... |
Detailed Description
Class containing the state of a download.libwww must be properly initialised before usage (this happens in glibwww-init.cc).
The purpose of this class is a minimal API for downloading HTTP and FTP URLs. A few useful extra features are added by Job::SingleURL.
The Output object is e.g. a GtkSingleUrl for single-file downloads
Constructor & Destructor Documentation
Download::Download | ( | const string & | uri, | |
Output * | o | |||
) |
Download::~Download | ( | ) |
References Assert, debug, and glibcurl_remove().
Member Function Documentation
void Download::init | ( | ) | [static] |
Initialize libwww - call this before starting any downloads.
References debug, glibcurl_init(), glibcurl_set_callback(), info, and JIGDO_VERSION.
Referenced by main().
void Download::cleanup | ( | ) | [static] |
Clean up - call this after all requests are finished.
References debug, and glibcurl_cleanup().
Referenced by main().
void Download::setResumeOffset | ( | uint64 | offset | ) | [inline] |
Set offset to resume from - download must not yet have been started, call before run().
Caution: The offset is not reset after the download has finished/failed and will be reused if you re-run(), so better always* call this immediately before run().
Referenced by Job::SingleUrl::setResumeOffset().
uint64 Download::resumeOffset | ( | ) | const [inline] |
void Download::run | ( | ) |
Whether to send a "Pragma: no-cache" header.
The header is sent iff pragmaNoCache==true. Caution: The setting is not reset after the download has finished/failed and will be reused if you re-run(), so better *always* call this immediately before run(). Start downloading. Returns (almost) immediately and runs the download in the background via the glib/libwww event loop. If resumeOffset > 0, continue downloading the data from a given byte position. If a resume is not possible (e.g. server does not support HTTP ranges), there's an error.
References Assert, curlDebug, curlWriter(), debug, glibcurl_add(), and resumeOffset().
Referenced by Job::SingleUrl::run().
const string & Download::uri | ( | ) | const [inline] |
Referenced by Job::SingleUrl::location().
void Download::pause | ( | ) |
Pause the request.
Actually, this only sets a flag, which causes the request to be paused at the next convenient opportunity. The socket of the Download is no longer passed to select().
References Assert, debug, and glibcurl_remove().
Referenced by Job::SingleUrl::pause().
void Download::cont | ( | ) |
Continue with the download.
References Assert, debug, glibcurl_add(), and paused().
Referenced by Job::SingleUrl::cont().
bool Download::paused | ( | ) | const [inline] |
Is the download paused? (i.e.
really paused now, not just pause() called.)
Referenced by cont(), and Job::SingleUrl::paused().
bool Download::failed | ( | ) | const [inline] |
Is the download paused, or is it not yet paused, but will be soon?
Did the download fail with an error?
Referenced by Job::SingleUrl::failed().
bool Download::succeeded | ( | ) | const [inline] |
Is download finished? (Also returns true if FTP/HTTP1.0 connection dropped).
Referenced by Job::SingleUrl::succeeded().
bool Download::interrupted | ( | ) | const [inline] |
The connection was interrupted during the download, or it timed out.
Referenced by Job::SingleUrl::resumePossible().
void Download::stop | ( | ) |
Forcibly stop this download.
Careful, in case we are pipelining it is unavoidable that other (pending and not pending) downloads in the same pipeline also fail; they'll have to be resumed. [outofdate:stop() must not be called when libwww is delivering data, i.e. you must not call it from your download_data() method.] stop() is special in that it neither calls job_failed() nor job_succeeded(). Usually, you will have to call one or the other (depending on context) to notify everyone that the request was stopped.
References Assert, debug, glibcurl_remove(), and NULL.
Referenced by Job::SingleUrl::stop().
Download::Output * Download::output | ( | ) | const [inline] |
Return the Output object.
void Download::setOutput | ( | Download::Output * | o | ) | [inline] |
Set the Output object.
The documentation for this class was generated from the following files:
- net/download.hh
- net/download.cc
Generated on Tue Sep 23 14:27:42 2008 for jigdo by
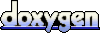