jigdo API
Last update by Admin on 2010-05-23
job/url-mapping.hh
Go to the documentation of this file.00001 /* $Id: url-mapping.hh,v 1.9 2005/04/10 17:04:04 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2004 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify it 00007 under the terms of the GNU General Public License, version 2. See the file 00008 COPYING for details. 00009 00010 */ 00019 #ifndef URL_MAPPING_HH 00020 #define URL_MAPPING_HH 00021 00022 #ifndef INLINE 00023 # ifdef NOINLINE 00024 # define INLINE 00025 # else 00026 # define INLINE inline 00027 # endif 00028 #endif 00029 00030 #include <config.h> 00031 00032 #include <string> 00033 #include <map> 00034 #include <memory> 00035 #include <set> 00036 #include <vector> 00037 00038 #include <debug.hh> 00039 #include <md5sum.hh> 00040 #include <nocopy.hh> 00041 #include <smartptr.hh> 00042 #include <status.hh> 00043 #include <uri.hh> /* for findLabelColon() */ 00044 #include <url-mapping.fh> 00045 //______________________________________________________________________ 00046 00053 class UrlMapping : public SmartPtrBase, public NoCopy { 00054 friend class ServerUrlMapping; 00055 friend class PartUrlMapping; 00056 public: 00058 static void setNoRandomInitialWeight(); 00059 00060 UrlMapping(); 00061 virtual ~UrlMapping() = 0; 00062 00065 inline void setUrl(const string& url, string::size_type pos = 0, 00066 string::size_type n = string::npos); 00068 inline const string& url() const { return urlVal; } 00069 00074 const char* parseOptions(const vector<string>& value); 00075 00079 inline void setPrepend(UrlMapping* um) { prepVal = um; } 00080 inline UrlMapping* prepend() const { return prepVal.get(); } 00081 00084 inline void insertNext(UrlMapping* um); 00086 inline UrlMapping* next() const { return nextVal.get(); } 00087 00089 bool empty() const { return url().empty() && prepend() == 0; } 00090 00100 static const double RANDOM_INIT_RANGE; 00101 00102 private: 00103 string urlVal; // Part of URL 00104 SmartPtr<UrlMapping> prepVal; // URL(s) to prepend to this one, or null 00105 SmartPtr<UrlMapping> nextVal; // Alt. to this mapping; singly linked list 00106 //LineInJigdoFilePointer def; // Definition of this mapping in .jigdo file 00107 00108 // Statistics, for server selection 00109 00110 // Nr of times we tried to download an URL generated using this mapping 00111 //unsigned tries; // 0 or 1 for PartUrlMapping, more possible for servers 00112 // Number of above tries which failed (404 not found, checksum error etc) 00113 //unsigned triesFailed; 00114 00115 /* Mapping-specific weight, includes user's global country preference, 00116 preference for this jigdo download's servers, global server preference. 00117 Does not change throughout the whole jigdo download. Can get <0. The 00118 higher the value, the higher the preference that will be given to this 00119 mapping. */ 00120 double weight; 00121 }; 00122 //______________________________________________________________________ 00123 00131 class ServerUrlMapping : public UrlMapping { 00132 // server-specific options: Supports resume, ... 00133 // server-specific availability counts 00134 }; 00135 //______________________________________________________________________ 00136 00142 class PartUrlMapping : public UrlMapping { 00143 public: 00150 string enumerate(vector<UrlMapping*>* best); 00151 00152 private: 00153 /* Because the UrlMapping data structure is not a tree, but an acyclic 00154 directed graph (i.e. tree with some branches coming together again), 00155 need to record the path through the structure while we recurse. */ 00156 struct StackEntry { 00157 UrlMapping* mapping; 00158 StackEntry* up; 00159 }; 00160 00161 void enumerate(StackEntry* stackPtr, UrlMapping* mapping, 00162 double score, unsigned pathLen, unsigned* serialNr, double* bestScore, 00163 vector<UrlMapping*>* bestPath, unsigned* bestSerialNr); 00164 00165 /* Set of URLs that were already returned by bestUnvisitedUrl(). Each URL 00166 is represented by a unique number, which is assigned to it by a 00167 depth-first scan of the tree-like structure in the UrlMap. */ 00168 auto_ptr<set<unsigned> > seen; 00169 }; 00170 //______________________________________________________________________ 00171 00174 class UrlMap : public NoCopy { 00175 public: 00176 inline UrlMap(); 00177 00183 const char* addPart(const string& baseUrl, const MD5& md, 00184 const vector<string>& value); 00185 00196 const char* addPart(const string& baseUrl, const vector<string>& value, 00197 SmartPtr<PartUrlMapping>* oldList); 00198 00205 const char* addServer(const string& baseUrl, const string& label, 00206 const vector<string>& value); 00207 00208 00210 void dumpJigdoInfo(); 00211 00212 /* [Parts] lines in .jigdo data; for each md5sum, there's a linked list of 00213 PartUrlMappings */ 00214 typedef map<MD5, SmartPtr<PartUrlMapping> > PartMap; 00215 /* [Servers] lines in .jigdo data; for each label string, there's a linked 00216 list of ServerUrlMappings */ 00217 typedef map<string, SmartPtr<ServerUrlMapping> > ServerMap; 00218 00219 const PartMap& parts() const { return partsVal; } 00220 const ServerMap& servers() const { return serversVal; } 00221 00223 inline PartUrlMapping* operator[](const MD5& m) const; 00224 00225 private: 00226 ServerUrlMapping* findOrCreateServerUrlMapping(const string& url, 00227 unsigned colon); 00228 PartMap partsVal; 00229 ServerMap serversVal; 00230 }; 00231 //====================================================================== 00232 00233 void UrlMapping::insertNext(UrlMapping* um) { 00234 Paranoid(um != 0 && um->nextVal.isNull()); 00235 um->nextVal = nextVal; 00236 nextVal = um; 00237 } 00238 00239 void UrlMapping::setUrl(const string& url, string::size_type pos, 00240 string::size_type n) { 00241 urlVal.assign(url, pos, n); 00242 } 00243 00244 UrlMap::UrlMap() : partsVal(), serversVal() { } 00245 00246 PartUrlMapping* UrlMap::operator[](const MD5& m) const { 00247 PartUrlMapping* result; 00248 PartMap::const_iterator i = parts().find(m); 00249 if (i != parts().end()) result = i->second.get(); else result = 0; 00250 return result; 00251 } 00252 00253 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
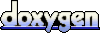