jigdo API
Last update by Admin on 2010-05-23
util/rsyncsum.hh
Go to the documentation of this file.00001 /* $Id: rsyncsum.hh,v 1.2 2003/09/27 21:31:04 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2000-2002 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00016 #ifndef RSYNCSUM_HH 00017 #define RSYNCSUM_HH 00018 00019 #ifndef INLINE 00020 # ifdef NOINLINE 00021 # define INLINE 00022 # else 00023 # define INLINE inline 00024 # endif 00025 #endif 00026 00027 #include <config.h> 00028 00029 #include <iosfwd> 00030 00031 #include <serialize.hh> 00032 //______________________________________________________________________ 00033 00047 class RsyncSum { 00048 public: 00050 RsyncSum() : sum(0) { }; 00052 RsyncSum(const byte* mem, size_t len) : sum(0) { addBack(mem, len); }; 00054 bool operator==(const RsyncSum& x) const { return get() == x.get(); } 00055 bool operator!=(const RsyncSum& x) const { return get() != x.get(); } 00056 bool operator< (const RsyncSum& x) const { return get() < x.get(); } 00057 bool operator> (const RsyncSum& x) const { return get() > x.get(); } 00058 bool operator<=(const RsyncSum& x) const { return get() <= x.get(); } 00059 bool operator>=(const RsyncSum& x) const { return get() >= x.get(); } 00061 RsyncSum& addBack(const byte* mem, size_t len); 00063 inline RsyncSum& addBack(byte x); 00068 inline RsyncSum& addBackNtimes(byte x, size_t n); 00075 RsyncSum& removeFront(const byte* mem, size_t len, size_t areaSize); 00077 inline RsyncSum& removeFront(byte x, size_t areaSize); 00079 uint32 get() const { return sum; } 00081 RsyncSum& reset() { sum = 0; return *this; } 00083 bool empty() const { return sum == 0; } 00084 // Using default dtor & copy ctor 00085 private: 00086 // Nothing magic about this constant; just a random number which, 00087 // according to the rsync sources, improves the quality of the 00088 // checksum. 00089 static const uint32 CHAR_OFFSET = 0xb593; 00090 uint32 sum; 00091 }; 00092 //________________________________________ 00093 00098 class RsyncSum64 { 00099 public: 00100 RsyncSum64() : sumLo(0), sumHi(0) { }; 00101 inline RsyncSum64(const byte* mem, size_t len); 00102 inline bool operator==(const RsyncSum64& x) const; 00103 inline bool operator!=(const RsyncSum64& x) const; 00104 inline bool operator< (const RsyncSum64& x) const; 00105 inline bool operator> (const RsyncSum64& x) const; 00106 inline bool operator<=(const RsyncSum64& x) const; 00107 inline bool operator>=(const RsyncSum64& x) const; 00108 INLINE RsyncSum64& addBack(const byte* mem, size_t len); 00109 INLINE RsyncSum64& addBack(byte x); 00110 INLINE RsyncSum64& addBackNtimes(byte x, size_t n); 00111 RsyncSum64& removeFront(const byte* mem, size_t len, size_t areaSize); 00112 inline RsyncSum64& removeFront(byte x, size_t areaSize); 00114 uint32 getLo() const { return sumLo; } 00116 uint32 getHi() const { return sumHi; } 00117 RsyncSum64& reset() { sumLo = sumHi = 0; return *this; } 00118 bool empty() const { return sumLo == 0 && sumHi == 0; } 00119 00120 template<class Iterator> 00121 inline Iterator serialize(Iterator i) const; 00122 template<class ConstIterator> 00123 inline ConstIterator unserialize(ConstIterator i); 00124 inline size_t serialSizeOf() const; 00125 00126 private: 00127 RsyncSum64& addBack2(const byte* mem, size_t len); 00128 static const uint32 charTable[256]; 00129 uint32 sumLo, sumHi; 00130 }; 00131 00132 INLINE ostream& operator<<(ostream& s, const RsyncSum& r); 00133 INLINE ostream& operator<<(ostream& s, const RsyncSum64& r); 00134 //______________________________________________________________________ 00135 00136 RsyncSum& RsyncSum::addBack(byte x) { 00137 uint32 a = sum; 00138 uint32 b = sum >> 16; 00139 a += x + CHAR_OFFSET; 00140 b += a; 00141 sum = ((a & 0xffff) + (b << 16)) & 0xffffffff; 00142 return *this; 00143 } 00144 00145 RsyncSum& RsyncSum::addBackNtimes(byte x, size_t n) { 00146 uint32 a = sum; 00147 uint32 b = sum >> 16; 00148 b += n * a + (n * (n + 1) / 2) * (x + CHAR_OFFSET); // Gauß 00149 a += n * (x + CHAR_OFFSET); 00150 sum = ((a & 0xffff) + (b << 16)) & 0xffffffff; 00151 return *this; 00152 } 00153 00154 RsyncSum& RsyncSum::removeFront(byte x, size_t areaSize) { 00155 uint32 a = sum; 00156 uint32 b = sum >> 16; 00157 b -= areaSize * (x + CHAR_OFFSET); 00158 a -= x + CHAR_OFFSET; 00159 sum = ((a & 0xffff) + (b << 16)) & 0xffffffff; 00160 return *this; 00161 } 00162 //________________________________________ 00163 00164 RsyncSum64::RsyncSum64(const byte* mem, size_t len) : sumLo(0), sumHi(0) { 00165 addBack(mem, len); 00166 }; 00167 00168 bool RsyncSum64::operator==(const RsyncSum64& x) const { 00169 return sumHi == x.sumHi && sumLo == x.sumLo; 00170 } 00171 bool RsyncSum64::operator!=(const RsyncSum64& x) const { 00172 return sumHi != x.sumHi || sumLo != x.sumLo; 00173 } 00174 bool RsyncSum64::operator< (const RsyncSum64& x) const { 00175 return (sumHi < x.sumHi) || (sumHi == x.sumHi && sumLo < x.sumLo); 00176 } 00177 bool RsyncSum64::operator> (const RsyncSum64& x) const { 00178 return (sumHi > x.sumHi) || (sumHi == x.sumHi && sumLo > x.sumLo); 00179 } 00180 bool RsyncSum64::operator<=(const RsyncSum64& x) const { 00181 return !(*this > x); 00182 } 00183 bool RsyncSum64::operator>=(const RsyncSum64& x) const { 00184 return !(*this < x); 00185 } 00186 00187 00188 RsyncSum64& RsyncSum64::removeFront(byte x, size_t areaSize) { 00189 sumHi = (sumHi - areaSize * charTable[x]) & 0xffffffff; 00190 sumLo = (sumLo - charTable[x]) & 0xffffffff; 00191 return *this; 00192 } 00193 00194 template<class Iterator> 00195 inline Iterator RsyncSum64::serialize(Iterator i) const { 00196 i = serialize4(sumLo, i); 00197 i = serialize4(sumHi, i); 00198 return i; 00199 } 00200 template<class ConstIterator> 00201 inline ConstIterator RsyncSum64::unserialize(ConstIterator i) { 00202 i = unserialize4(sumLo, i); 00203 i = unserialize4(sumHi, i); 00204 return i; 00205 } 00206 inline size_t RsyncSum64::serialSizeOf() const { return 8; } 00207 00208 #ifndef NOINLINE 00209 # include <rsyncsum.ih> /* NOINLINE */ 00210 #endif 00211 00212 #endif
Generated on Tue Sep 23 14:27:42 2008 for jigdo by
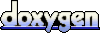