jigdo API
Last update by Admin on 2010-05-23
job/job.hh
Go to the documentation of this file.00001 /* $Id: job.hh,v 1.4 2003/09/16 23:32:10 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2003 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00018 #ifndef JOB_HH 00019 #define JOB_HH 00020 00021 #include <config.h> 00022 00023 #include <debug.hh> 00024 #include <ilist.hh> 00025 00026 #include <string> 00027 //______________________________________________________________________ 00028 00029 namespace Job { 00030 class IO; 00031 } 00032 //______________________________________________________________________ 00033 00034 //#define _DEPRECATED __attribute__((deprecated)) 00035 //#define _DEPRECATED 00036 00053 class Job::IO : public IListBase { 00054 public: 00055 00056 virtual ~IO() { } 00057 00059 void removeListener() { iList_remove(); } 00060 00064 virtual void job_deleted() = 0; 00065 00067 virtual void job_succeeded() = 0; 00068 00071 virtual void job_failed(const string& message) = 0; 00072 00074 virtual void job_message(const string& message) = 0; 00075 }; 00076 //______________________________________________________________________ 00077 00078 // For IOSource<SomeIO> io, use 00079 // IOSOURCE_SEND(SomeIO, io, job_failed, ("it failed")); 00080 // It is OK if any called object deletes itself in response to the call 00081 #define IOSOURCE_SEND(_ioClass, _ioObj, _functionName, _args) \ 00082 do { \ 00083 IList<_ioClass>& _listeners = (_ioObj).listeners(); \ 00084 IList<_ioClass>::iterator _i = _listeners.begin(), _e = _listeners.end(); \ 00085 while (_i != _e) { \ 00086 _ioClass* _listObj = &*_i; ++_i; _listObj->_functionName _args; \ 00087 } \ 00088 } while (false) 00089 // Same thing, but const: 00090 #define IOSOURCE_SENDc(_ioClass, _ioObj, _functionName, _args) \ 00091 do { \ 00092 const IList<_ioClass>& _listeners = (_ioObj).listeners(); \ 00093 IList<_ioClass>::const_iterator _i = _listeners.begin(), \ 00094 _e = _listeners.end(); \ 00095 while (_i != _e) { \ 00096 _ioClass* _listObj = &*_i; ++_i; _listObj->_functionName _args; \ 00097 } \ 00098 } while (false) 00099 // Same thing, but for template function 00100 #define IOSOURCE_SENDt(_ioClass, _ioObj, _functionName, _args) \ 00101 do { \ 00102 IList<_ioClass>& _listeners = (_ioObj).listeners(); \ 00103 typename IList<_ioClass>::iterator _i = _listeners.begin(), \ 00104 _e = _listeners.end(); \ 00105 while (_i != _e) { \ 00106 _ioClass* _listObj = &*_i; ++_i; _listObj->_functionName _args; \ 00107 } \ 00108 } while (false) 00109 //________________________________________ 00110 00121 template<class SomeIO> 00122 class IOSource : NoCopy { 00123 public: 00124 IOSource() : list() { } 00126 ~IOSource() { IOSOURCE_SENDt(SomeIO, *this, job_deleted, ()); } 00130 void addListener(SomeIO& l) { Assert(&l != 0); list.push_front(l); } 00131 const IList<SomeIO>& listeners() const { return list; }; 00132 IList<SomeIO>& listeners() { return list; }; 00133 bool empty() const { return list.empty(); } 00134 00135 private: 00136 IList<SomeIO> list; 00137 }; 00138 //______________________________________________________________________ 00139 00140 #if 0 00141 template<class SomeIO> 00142 class IOPtr { 00143 public: 00144 inline IOPtr(SomeIO* io) _DEPRECATED; 00145 ~IOPtr() { if (ptr != 0) ptr->job_deleted(); } 00146 00147 SomeIO& operator*() { return *ptr; } 00148 SomeIO* operator->() { return ptr; } 00149 operator bool() { return ptr != 0; } 00150 SomeIO* get() { return ptr; } 00152 void set(SomeIO* io) { 00153 Paranoid(ptr == 0); 00154 ptr = io; 00155 } 00157 void remove(SomeIO* rmIo) { 00158 Paranoid(ptr != 0); 00159 Job::IO* newPtr = ptr->job_removeIo(rmIo); 00160 // Upcast is OK if the job_removeIo() implementation plays by the rules 00161 Paranoid(newPtr == 0 || dynamic_cast<SomeIO*>(newPtr) != 0); 00162 ptr = static_cast<SomeIO*>(newPtr); 00163 } 00164 00166 void release() { ptr = 0; } 00167 00168 private: 00169 SomeIO* ptr; 00170 }; 00171 00172 template<class SomeIO> 00173 IOPtr<SomeIO>::IOPtr(SomeIO* io) : ptr(io) { } 00174 #endif 00175 00176 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
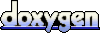