jigdo API
Last update by Admin on 2010-05-23
jigdoconfig.hh
Go to the documentation of this file.00001 /* $Id: jigdoconfig.hh,v 1.1.1.1 2003/07/04 22:29:17 atterer Exp $ -*- C++ -*- 00002 __ _ 00003 |_) /| Copyright (C) 2001-2002 | richard@ 00004 | \/¯| Richard Atterer | atterer.net 00005 ¯ '` ¯ 00006 This program is free software; you can redistribute it and/or modify 00007 it under the terms of the GNU General Public License, version 2. See 00008 the file COPYING for details. 00009 00010 */ 00020 #ifndef JIGDOCONFIG_HH 00021 #define JIGDOCONFIG_HH 00022 00023 #include <map> 00024 #include <string> 00025 #include <vector> 00026 00027 #include <configfile.hh> 00028 //______________________________________________________________________ 00029 00031 class JigdoConfig { 00032 private: 00033 /* multimap doesn't make guarantees about order of inserted values 00034 with equal key, which needs to be preserved in our case. */ 00035 typedef map<string,vector<string> > Map; 00036 //________________________________________ 00037 00038 public: 00041 class ProgressReporter { 00042 public: 00043 virtual ~ProgressReporter() { } 00044 virtual void error(const string& message); 00045 virtual void info(const string& message); 00046 }; 00047 //________________________________________ 00048 00049 #if 0 /* Use same function from net/uri.hh */ 00050 00054 static inline unsigned findLabelColon(const string& s); 00055 #endif 00056 //________________________________________ 00057 00059 JigdoConfig(const char* jigdoFile, ProgressReporter& pr); 00060 JigdoConfig(const string& jigdoFile, ProgressReporter& pr); 00064 JigdoConfig(const char* jigdoFile, ConfigFile* configFile, 00065 ProgressReporter& pr); 00066 JigdoConfig(const string& jigdoFile, ConfigFile* configFile, 00067 ProgressReporter& pr); 00068 ~JigdoConfig() { delete config; } 00069 ConfigFile& configFile() { return *config; } 00070 //________________________________________ 00071 00076 void rescan(); 00077 00079 inline void setReporter(ProgressReporter& pr); 00080 00089 class Lookup { 00090 public: 00091 inline Lookup(const JigdoConfig& jc, const string& query); 00095 inline bool next(string& result); 00096 // Default copy ctor and dtor 00097 private: 00098 const JigdoConfig& config; 00099 // "MyServer:dir/foo/file.gz" or null: next() returns false 00100 const string* uri; 00101 string::size_type colon; // Offset of ':' in query 00102 // Pointer in list for "MyServer" mappings; pointer to end of list 00103 vector<string>::const_iterator cur, end; 00104 }; 00105 friend class Lookup; 00106 //________________________________________ 00107 00108 private: 00109 00110 /* Adds filename and line number before reporting. This is used by 00111 JigdoConfig to talk to ConfigFile. */ 00112 struct ForwardReporter : ConfigFile::ProgressReporter { 00113 inline ForwardReporter(JigdoConfig::ProgressReporter& pr, 00114 const string& file); 00115 inline ForwardReporter(JigdoConfig::ProgressReporter& pr, 00116 const char* file); 00117 virtual ~ForwardReporter() { } 00118 virtual void error(const string& message, const size_t lineNr = 0); 00119 virtual void info(const string& message, const size_t lineNr = 0); 00120 JigdoConfig::ProgressReporter* reporter; 00121 string fileName; 00122 }; 00123 //________________________________________ 00124 00125 struct ServerLine { 00126 ConfigFile::iterator line; 00127 size_t labelStart, labelEnd, valueStart; 00128 }; 00129 Map::iterator rescan_addLabel(list<ServerLine>& entries, 00130 const string& label, bool& printError); 00131 inline void rescan_makeSubst(list<ServerLine>& entries, Map::iterator mapl, 00132 const ServerLine& l, bool& printError); 00133 void scanVersionInfo(); // Check for supported file format version number 00134 00135 ConfigFile* config; 00136 Map serverMap; 00137 ForwardReporter freporter; 00138 }; 00139 //______________________________________________________________________ 00140 00141 JigdoConfig::ForwardReporter::ForwardReporter( 00142 JigdoConfig::ProgressReporter& pr, const string& file) 00143 : reporter(&pr), fileName(file) { } 00144 00145 JigdoConfig::ForwardReporter::ForwardReporter( 00146 JigdoConfig::ProgressReporter& pr, const char* file) 00147 : reporter(&pr), fileName(file) { } 00148 00149 void JigdoConfig::setReporter(ProgressReporter& pr) { 00150 freporter.reporter = ≺ 00151 } 00152 //________________________________________ 00153 00154 JigdoConfig::Lookup::Lookup(const JigdoConfig& jc, const string& query) 00155 : config(jc), uri(&query), colon(query.find(':')), cur() { 00156 /* colon == string::npos means: The URI doesn't contain a ':', or it 00157 does but the label before it ("MyServer") isn't listed in the 00158 mapping, or the label is listed but the corresponding 00159 vector<string> is empty. In all these cases, the Lookup will only 00160 return one string - the original query. */ 00161 if (colon != string::npos) { 00162 string label(query, 0, colon); 00163 Map::const_iterator vec = config.serverMap.find(label); 00164 ++colon; 00165 if (vec != config.serverMap.end() && vec->second.size() != 0) { 00166 cur = vec->second.begin(); 00167 end = vec->second.end(); 00168 } else { 00169 colon = string::npos; 00170 } 00171 } 00172 } 00173 00174 bool JigdoConfig::Lookup::next(string& result) { 00175 if (uri == 0) return false; 00176 if (colon == string::npos) { // Only return one value 00177 result = *uri; 00178 uri = 0; 00179 return true; 00180 } 00181 // Iterate through vector 00182 result = *cur; 00183 result.append(*uri, colon, string::npos); 00184 ++cur; 00185 if (cur == end) uri = 0; 00186 return true; 00187 } 00188 00189 #endif
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
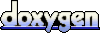