jigdo API
Last update by Admin on 2010-05-23
util/glibc-getopt.h
Go to the documentation of this file.00001 /* Taken from glibc 2.1.3 */ 00002 00003 /* Declarations for getopt. 00004 Copyright (C) 1989,90,91,92,93,94,96,97,98 Free Software Foundation, Inc. 00005 This file is part of the GNU C Library. 00006 00007 The GNU C Library is free software; you can redistribute it and/or 00008 modify it under the terms of the GNU Library General Public License as 00009 published by the Free Software Foundation; either version 2 of the 00010 License, or (at your option) any later version. 00011 00012 The GNU C Library is distributed in the hope that it will be useful, 00013 but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 Library General Public License for more details. 00016 00017 You should have received a copy of the GNU Library General Public 00018 License along with the GNU C Library; see the file COPYING.LIB. If not, 00019 write to the Free Software Foundation, Inc., 59 Temple Place - Suite 330, 00020 Boston, MA 02111-1307, USA. */ 00021 00022 #ifndef GLIBC_GETOPT_H 00023 00024 #ifndef __need_getopt 00025 # define GLIBC_GETOPT_H 1 00026 #endif 00027 00028 #include <config.h> 00029 #if HAVE_GETOPT_LONG 00030 # include <getopt.h> 00031 # define ELIDE_CODE 00032 #else 00033 /* RA: Need this for statically linked binaries on Solaris */ 00034 #define opterr my_opterr 00035 #define optind my_optind 00036 #define optopt my_optopt 00037 00038 #ifdef __cplusplus 00039 extern "C" { 00040 #endif 00041 00042 /* For communication from `getopt' to the caller. 00043 When `getopt' finds an option that takes an argument, 00044 the argument value is returned here. 00045 Also, when `ordering' is RETURN_IN_ORDER, 00046 each non-option ARGV-element is returned here. */ 00047 00048 extern char *optarg; 00049 00050 /* Index in ARGV of the next element to be scanned. 00051 This is used for communication to and from the caller 00052 and for communication between successive calls to `getopt'. 00053 00054 On entry to `getopt', zero means this is the first call; initialize. 00055 00056 When `getopt' returns -1, this is the index of the first of the 00057 non-option elements that the caller should itself scan. 00058 00059 Otherwise, `optind' communicates from one call to the next 00060 how much of ARGV has been scanned so far. */ 00061 00062 extern int optind; 00063 00064 /* Callers store zero here to inhibit the error message `getopt' prints 00065 for unrecognized options. */ 00066 00067 extern int opterr; 00068 00069 /* Set to an option character which was unrecognized. */ 00070 00071 extern int optopt; 00072 00073 #ifndef __need_getopt 00074 /* Describe the long-named options requested by the application. 00075 The LONG_OPTIONS argument to getopt_long or getopt_long_only is a vector 00076 of `struct option' terminated by an element containing a name which is 00077 zero. 00078 00079 The field `has_arg' is: 00080 no_argument (or 0) if the option does not take an argument, 00081 required_argument (or 1) if the option requires an argument, 00082 optional_argument (or 2) if the option takes an optional argument. 00083 00084 If the field `flag' is not NULL, it points to a variable that is set 00085 to the value given in the field `val' when the option is found, but 00086 left unchanged if the option is not found. 00087 00088 To have a long-named option do something other than set an `int' to 00089 a compiled-in constant, such as set a value from `optarg', set the 00090 option's `flag' field to zero and its `val' field to a nonzero 00091 value (the equivalent single-letter option character, if there is 00092 one). For long options that have a zero `flag' field, `getopt' 00093 returns the contents of the `val' field. */ 00094 00095 struct option 00096 { 00097 # if defined __STDC__ && __STDC__ 00098 const char *name; 00099 # else 00100 char *name; 00101 # endif 00102 /* has_arg can't be an enum because some compilers complain about 00103 type mismatches in all the code that assumes it is an int. */ 00104 int has_arg; 00105 int *flag; 00106 int val; 00107 }; 00108 00109 /* Names for the values of the `has_arg' field of `struct option'. */ 00110 00111 # define no_argument 0 00112 # define required_argument 1 00113 # define optional_argument 2 00114 #endif /* need getopt */ 00115 00116 00117 /* Get definitions and prototypes for functions to process the 00118 arguments in ARGV (ARGC of them, minus the program name) for 00119 options given in OPTS. 00120 00121 Return the option character from OPTS just read. Return -1 when 00122 there are no more options. For unrecognized options, or options 00123 missing arguments, `optopt' is set to the option letter, and '?' is 00124 returned. 00125 00126 The OPTS string is a list of characters which are recognized option 00127 letters, optionally followed by colons, specifying that that letter 00128 takes an argument, to be placed in `optarg'. 00129 00130 If a letter in OPTS is followed by two colons, its argument is 00131 optional. This behavior is specific to the GNU `getopt'. 00132 00133 The argument `--' causes premature termination of argument 00134 scanning, explicitly telling `getopt' that there are no more 00135 options. 00136 00137 If OPTS begins with `--', then non-option arguments are treated as 00138 arguments to the option '\0'. This behavior is specific to the GNU 00139 `getopt'. */ 00140 00141 #if defined __STDC__ && __STDC__ 00142 # ifdef __GNU_LIBRARY__ 00143 /* Many other libraries have conflicting prototypes for getopt, with 00144 differences in the consts, in stdlib.h. To avoid compilation 00145 errors, only prototype getopt for the GNU C library. */ 00146 extern int getopt (int __argc_, char *const *__argv_, const char *__shortopts); 00147 # else /* not __GNU_LIBRARY__ */ 00148 /* [RA] breaks on Solaris: extern int getopt (); */ 00149 # endif /* __GNU_LIBRARY__ */ 00150 00151 # ifndef __need_getopt 00152 extern int getopt_long (int __argc_, char *const *__argv_, const char *__shortopts, 00153 const struct option *__longopts, int *__longind); 00154 extern int getopt_long_only (int __argc_, char *const *__argv_, 00155 const char *__shortopts, 00156 const struct option *__longopts, int *__longind); 00157 00158 /* Internal only. Users should not call this directly. */ 00159 extern int _getopt_internal (int __argc_, char *const *__argv_, 00160 const char *__shortopts, 00161 const struct option *__longopts, int *__longind, 00162 int __long_only); 00163 # endif 00164 #else /* not __STDC__ */ 00165 extern int getopt (); 00166 # ifndef __need_getopt 00167 extern int getopt_long (); 00168 extern int getopt_long_only (); 00169 00170 extern int _getopt_internal (); 00171 # endif 00172 #endif /* __STDC__ */ 00173 00174 #ifdef __cplusplus 00175 } 00176 #endif 00177 00178 /* Make sure we later can get all the definitions and declarations. */ 00179 #undef __need_getopt 00180 00181 #endif /* HAVE_GETOPT_LONG */ 00182 00183 #endif /* getopt.h */
Generated on Tue Sep 23 14:27:41 2008 for jigdo by
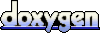